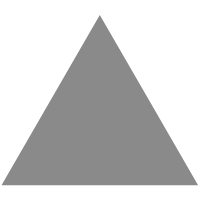
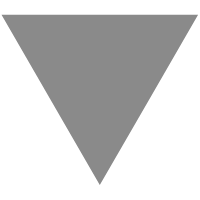
通过事例重温一下 JS 中 常见的15 种数组操作(备忘清单)
source link: https://juejin.im/post/5ddc6ad8518825731161fa75
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
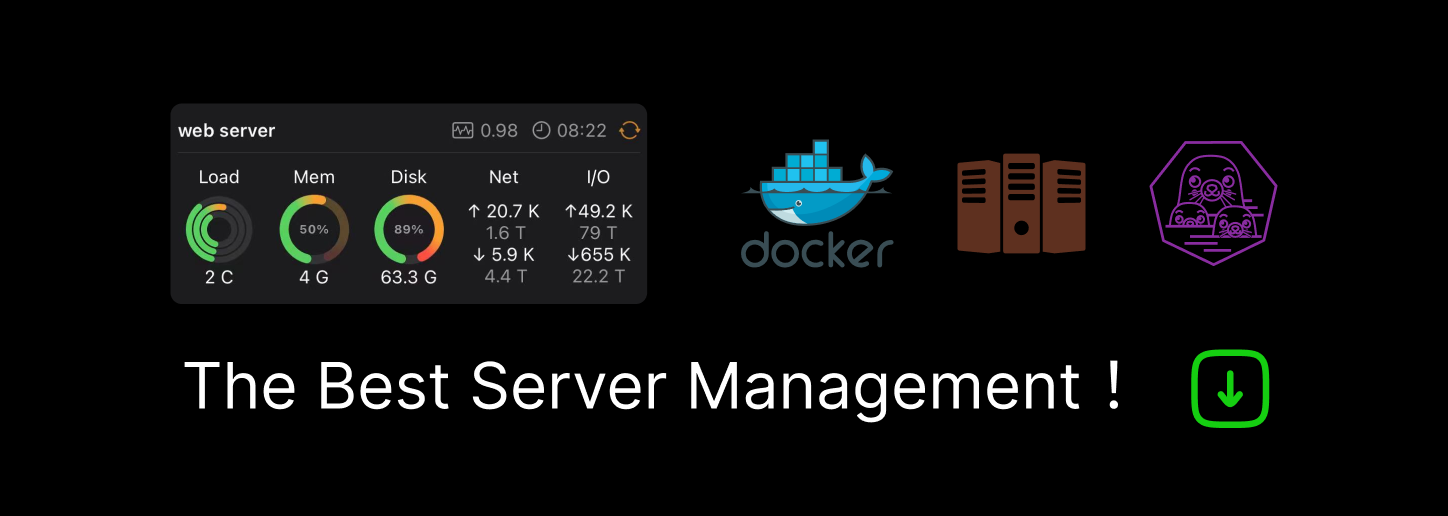
通过事例重温一下 JS 中 常见的15 种数组操作(备忘清单)
作者:Dmitri Pavlutin
译者:前端小智
来源:dmitripavlutin.com
你知道的越多,你不知道的越多
点赞再看,养成习惯
本文 GitHub:github.com/qq449245884… 上已经收录,更多往期高赞文章的分类,也整理了很多我的文档,和教程资料。欢迎Star和完善,大家面试可以参照考点复习,希望我们一起有点东西。
数组是 JS 中广泛使用的数据结构。数组对象提供了大量有用的方法,如array. forEach()
、array.map()
等来操作数组。
在实战中,我经常对数组可能的操作和相应采用哪个更好的方法不知所措,所以本文就列出 15
种常用数据方法,让咱们重温加强记忆一下。
1. 数组的遍历
1.1 for..of
循环
for(const item of items)
循环遍历数组项,如下所示遍历colors
列表:
const colors = ['blue', 'green', 'white'];
for (const color of colors) {
console.log(color);
}
// 'blue'
// 'green'
// 'white'
复制代码
提示:
咱们可以随时使用break
语句停止遍历。
1.2 for
循环
for(let i; i < array.length; i++)
循环使用递增的索引变量遍历数组项。
for
通常需要在每个循环中递增index
变量
const colors = ['blue', 'green', 'white'];
for (let index = 0; index < colors.length; index++) {
const color = colors[index];
console.log(color);
}
// 'blue'
// 'green'
// 'white'
复制代码
index
变量从0
递增到colors.length-1
。此变量用于按以下索引访问项:colors [index]
。
提示
咱们可以随时使用break
语句停止遍历。
1.3 array.forEach()
方法
array.forEach(callback)
方法通过在每个数组项上调用callback
函数来遍历数组项。
在每次遍历中,都使用以下参数调用callback(item [, index [, array]])
:当前遍历项,当前遍历索引和数组本身。
const colors = ['blue', 'green', 'white'];
colors.forEach(function callback(value, index) {
console.log(value, index);
});
// 'blue', 0
// 'green', 1
// 'white', 2
复制代码
提示:
咱们不能中断array.forEach()
迭代。
2. 数组的映射
2.1 Array.map()
方法
array.map(callback)
方法通过在每个数组项上使用callback
调用结果来创建一个新数组。
在每个遍历中的callback(item[, index[, array]])
使用参数调用:当前项、索引和数组本身,并应该返回新项。
如下所示咱们对每个数组元素都递增 1
:
const numbers = [0, 2, 4];
const newNumbers = numbers.map(function increment(number) {
return number + 1;
});
newNumbers; // => [1, 3, 5]
复制代码
提示:
array.map()
创建一个新的映射数组,而不改变原始数组。
2.2 Array.from()
方法
Array.from(arrayLike[, callback])
方法通过在每个数组项上使用callback
调用结果来创建一个新数组。
在每个遍历中callback(item[, index[, array]])
使用参数调用:当前项、索引和数组本身并且应该返回新项。
如下所示咱们对每个数组元素都递增 1
:
const numbers = [0, 2, 4];
const newNumbers = Array.from(numbers,
function increment(number) {
return number + 1;
}
);
newNumbers; // => [1, 3, 5]
复制代码
提示:
Array.from()
创建一个新的映射数组,而不改变原始数组。Array.from()
更适合从类似数组的对象进行映射。
3. 数据的简化
3.1 Array.reduce()
方法
array.reduce(callback[, initialValue])
通过调用callback
函数来将数组简化为一个值。
在每次遍历中的callback(accumulator, item[, index[, array]])
使用用参数调用的:累加器,当前项,索引和数组本身且应该返回累加器。
经典示例是对数字数组求和:
const numbers = [2, 0, 4];
function summarize(accumulator, number) {
return accumulator + number;
}
const sum = numbers.reduce(summarize, 0);
sum; // => 6
复制代码
第一步,将accumulator
初始化为0
。然后,对每个累加数字和的数组项调用summary
函数。
提示:
如果没有使用 initialValue 来设置初始值,则默认使用数组的第一个元素作为初始值。
4. 数据的连接
4.1 array.concat()
方法
array.concat(array1[, array2, ...])
将一个或多个数组连接到原始数组。如下所示,连接两个数组:
const heroes = ['小智', '前端小智'];
const villains = ['老王', '小三'];
const everyone = heroes.concat(villains);
everyone // ["小智", "前端小智", "老王", "小三"]
复制代码
提示:
concat()
创建一个新的数组,而不改变原来的数组array.concat(array1 [,array2,...])
接受多个要连接的数组。
4.2 展开操作符号
咱们将展开操作符与数组字面量一起使用来连接数组:[...array1, ...array2]
。
const heroes = ['小智', '前端小智'];
const villains = ['老王', '小三'];
const names = [...heroes, ...villains];
names; // ["小智", "前端小智", "老王", "小三"]
复制代码
提示:
[...arr1, ...arr2, ...arrN]
:咱们可以使用展开运算符连接所需数量的数组。
获取数组的片段
5.1 array.slice()
方法
array.slice([fromIndex [,toIndex]])
返回数组的一个片段,该片段从fromIndex
开始,以toIndex
结尾(不包括toIndex
本身)。fromIndex
可选参数默认为0
,toIndex
可选参数默认为array.length
。
const names = ["小智", "前端小智", "老王", "小三"]
const heroes = names.slice(0, 2)
const villains = names.splice(2)
heroes // ["小智", "前端小智"]
villains // ["老王", "小三"]
复制代码
提示:
array.slice()
创建一个新数组,而不改变原始数组。
6. 数组的拷贝
6.1 展开操作符
拷贝数组的一种简单方法是使用展开运算符:const clone = [... array]
,如下所示,拷贝 colors
数组:
const colors = ['white', 'black', 'gray'];
const clone = [...colors];
clone; // => ['white', 'black', 'gray']
colors === clone; // => false
复制代码
[...array]
创建一个浅拷贝。
6.2 array.concat()
方法
[].concat(array)
是另一种拷贝数组的方法。
const colors = ['white', 'black', 'gray'];
const clone = [].concat(colors);
clone; // => ['white', 'black', 'gray']
colors === clone; // => false
复制代码
[].concat(array)
创建一个浅拷贝。
6.3 array.slice()
方法
array.slice())
是另一种拷贝数组的方法。
const colors = ['white', 'black', 'gray'];
const clone = colors.slice();
clone; // => ['white', 'black', 'gray']
colors === clone; // => false
复制代码
colors.slice()
创建一个浅拷贝。
7. 查找数组
7.1 array.includes()
方法
array.includes(itemToSearch [,fromIndex])
返回一个布尔值,array
是否包含itemToSearch
。 可选参数fromIndex
,默认为0
,表示开始搜索的索引。如下所示:判断2
和99
是否存在于一组数字中:
const numbers = [1, 2, 3, 4, 5];
numbers.includes(2); // => true
numbers.includes(99); // => false
复制代码
7.2 array.find()
方法
array.find(predicate)
方法返回数组中满足提供的测试函数的第一个元素的值。否则返回 undefined
。
如下所示,找到数组中的第一个偶数:
const numbers = [1, 2, 3, 4, 5];
function isEven(number) {
return number % 2 === 0;
}
const evenNumber = numbers.find(isEven);
evenNumber; // => 2
复制代码
7.3 array.indexOf()
方法
array.indexOf(itemToSearch[, fromIndex])
返回array
中第一个出现的itemToSearch
的索引。默认为0的
可选参数fromIndex
表示开始搜索的索引。
如下所示,找到前端小智
的索引:
const names = ["小智", "前端小智", "老王", "小三"]
const index = names.indexOf('前端小智')
index // 1
复制代码
提示:
- 如果找不到该项,则
array.indexOf(itemToSearch)
返回-1
array.findIndex(predicate)
是使用predicate
函数查找索引的替代方法。
8. 查询数组
8.1 array.every()
方法
如果每个项都通过predicate
检查,则array.every(predicate)
返回true
。
在每个遍历predicate(item[, index[, array]])
上,用参数调用predicate
函数:当前遍历项、索引和数组本身。
如下所示,确定数组是否只包含偶数:
const evens = [0, 2, 4, 6];
const numbers = [0, 1, 4, 6];
function isEven(number) {
return number % 2 === 0;
}
evens.every(isEven); // => true
numbers.every(isEven); // => false
复制代码
8.2 array.some()
方法
如果每个项只要一个通过predicate
检查,则array.every(predicate)
返回true
。
在每个遍历predicate(item[, index[, array]])
上,用参数调用predicate
函数:当前遍历项、索引和数组本身。
如下所示:确定数组是否至少包含一个偶数:
const numbers = [1, 5, 7, 10];
const odds = [1, 3, 3, 3];
function isEven(number) {
return number % 2 === 0;
}
numbers.some(isEven); // => true
odds.some(isEven); // => false
复制代码
9. 数组的过滤
9.1 array.filter()
方法
array.filter(predicate)
方法创建一个新数组, 其包含通过所提供函数实现的测试的所有元素。
在每个遍历predicate(item[, index[, array]])
上,用参数调用predicate
函数:当前遍历项、索引和数组本身。
如下所示:将一个数组过滤为仅包含偶数:
const numbers = [1, 5, 7, 10];
function isEven(number) {
return number % 2 === 0;
}
const evens = numbers.filter(isEven);
evens; // => [10]
复制代码
提示:
array.filter()
创建一个新数组,而不改变原始数组。
10. 数组的插入
10.1 array.push()
方法
array.push(item1 [...,itemN])
方法将一个或多个项追加到数组的末尾,并返回新的长度。
如下所示,在names
数组的末尾添加 '小智'
const names = ['小智']
names.push('前端小智')
names // ["小智", "前端小智"]
复制代码
提示:
array.push()
会改变原数组array.push(item1, item2, ..., itemN)
可以添加多个元素。
10.2 array.unshift()
方法
array.unshift(item1[..., itemN])
方法将一个或多个项追加到数组的开头,返回数组的新长度
const names = ['小智']
names.unshift('前端小智')
names // ["前端小智", "小智"]
复制代码
提示:
array.unshift()
会改变原数组array.unshift(item1, item2, ..., itemN)
可以添加多个元素。
10.3 展开操作符
可以通过组合展开操作符和数组字面量以不可变的方式在数组中插入项。
在数组末尾追加一个项:
const names = ['小智', '大治']
const names2 = [...names, '王大冶']
names2 // ["小智", "大治", "王大冶"]
复制代码
在数组的开头追加一个项:
const names = ['小智', '大治']
const names2 = [
'王大冶',
...names
]
names2 // ["王大冶", "小智", "大治"]
复制代码
在任何索引处插入元素:
const names = ['小智', '大治']
const indexToInsert = 1
const names2 = [
...names.slice(0, indexToInsert),
'前端小智',
...names.slice(indexToInsert)
]
names2 // ["小智", "前端小智", "大治"]
复制代码
11. 删除数组元素
11.1 array.pop()
方法
array.pop()
方法从数组中删除最后一个元素,然后返回该元素。如下所示,删除colors
数组的最后一个元素:
const colors = ['blue', 'green', 'black'];
const lastColor = colors.pop();
lastColor; // => 'black'
colors; // => ['blue', 'green']
复制代码
array.pop()
会改变原数组。
11.2 array.shift()
方法
array.shift()
方法从数组中删除第一个元素,然后返回该元素。
const colors = ['blue', 'green', 'black'];
const firstColor = colors.shift();
firstColor; // => 'blue'
colors; // => ['green', 'black']
复制代码
-
array.shift()
会改变原数组。 -
array.shift()
具有O(n)
复杂度。
11.3 array.splice()
方法
array.splice(fromIndex[, removeCount[, item1[, item2[, ...]]]])
从数组中删除元素,并插入新的元素。
例如,咱们从索引1
处删除2
个元素:
const names = ['张三', '李四', '王五', '赵六']
names.splice(1, 2)
names // => ["张三", "赵六"]
复制代码
names.splice(1,2)
删除元素'张三'
和'赵六'
。
names.splice()
可以插入新元素,而不是插入已删除的元素。 咱们可以替换索引1
处开始的的2
个元素,然后插入一个新的元素 '小智'
:
const names = ['张三', '李四', '王五', '赵六']
names.splice(1, 2, '小智')
names // ["张三", "小智", "赵六"]
复制代码
array.splice()
会改变原数组。
11.4 展开操作符号
可以通过组合展开操作符和数据字面量以不可变的方式从数组中删除项。
const names = ['张三', '李四', '王五', '赵六']
const fromIndex = 1
const removeCount = 2
const newNames = [
...names.slice(0, fromIndex),
...names.slice(fromIndex + removeCount)
]
newNames // ["张三", "赵六"]
复制代码
12. 清空数组
12.1 array.length
属性
array.length
是保存数组长度的属性。 除此之外,array.length
是可写的。
如果咱们写一个小于当前长度的array.length = newLength
,多余的元素从数组中移除。
如下所示:使用array.length = 0
删除数组中的所有项目:
const colors = ['blue', 'green', 'black'];
colors.length = 0;
colors; // []
复制代码
12.2 array.splice()
方法
array.splice(fromIndex[, removeCount[, item1[, item2[, ...]]]])
从数组中删除元素,并插入新的元素。
如果removeCount
参数被省略,那么array.splice()
将删除从fromIndex
开始的数组的所有元素。咱们使用它来删除数组中的所有元素:
const colors = ['blue', 'green', 'black'];
colors.splice(0);
colors; // []
复制代码
13. 填充数组
13.1 array.fill()
方法
array.fill(value[, fromIndex[, toIndex]])
用从fromIndex
到toIndex
的值填充数组(不包括toIndex
本身)。fromIndex
可选参数默认为0
,toIndex
可选参数默认为array.length
。
例如,使用用零值填充数组:
const numbers = [1, 2, 3, 4];
numbers.fill(0);
numbers; // => [0, 0, 0, 0]
复制代码
不仅如此,还可以使用Array(length).fill(initial)
来初始化特定长度和初始值的数组。
const length = 3;
const zeros = Array(length).fill(0);
zeros; // [0, 0, 0]
复制代码
array.fill()
会改变原数组。
13.2 Array.from()
函数
Array.from()
有助于初始化带有对象的特定长度的数组:
const length = 4;
const emptyObjects = Array.from(Array(length), function() {
return {};
});
emptyObjects; // [{}, {}, {}, {}]
复制代码
14. 数组的扁平化
14.1 array.flat()
方法
array.flat([depth])
方法通过递归扁平属于数组的项直到一定深度来创建新数组。 depth
可选参数默认为1
:
const arrays = [0, [1, 3, 5], [2, 4, 6]];
const flatArray = arrays.flat();
flatArray; // [0, 1, 3, 5, 2, 4, 6]
复制代码
arrays
包含数字和数字数组的混合。 arrays.flat()
对数组进行扁平,使其仅包含数字。
提示:
array.flat()
创建一个新数组,而不会改变原始数组。
15. 数组的排序
15.1 array.sort()
方法
array.sort([compare])
方法对数组的元素进行排序。
可选参数compare(a, b)
是一个自定义排序顺的回调函数。如果比较compare(a, b)
返回的结果:
- 如果
a
小于b
,在排序后的数组中a
应该出现在b
之前,就返回一个小于0
的值。 - 如果
a
等于b
,就返回0
。 - 如果
a
大于b
,就返回一个大于0
的值。
如下所示,对数组 numbers
时行排序
const numbers = [4, 3, 1, 2];
numbers.sort();
numbers; // => [1, 2, 3, 4]
复制代码
numbers.sort()
以升序对数字进行排序。
使用比较函数,让偶数排在奇数前面:
const numbers = [4, 3, 1, 2];
function compare(n1, n2) {
if (n1 % 2 === 0 && n2 % 2 !== 0) {
return -1;
}
if (n1 % 2 !== 0 && n2 % 2 === 0) {
return 1;
}
return 0;
}
numbers.sort(compare);
numbers; // => [4, 2, 3, 1]
复制代码
提示:
array.sort()
会改变原数组。
原文:dmitripavlutin.com/operations-…
代码部署后可能存在的BUG没法实时知道,事后为了解决这些BUG,花了大量的时间进行log 调试,这边顺便给大家推荐一个好用的BUG监控工具 Fundebug。
干货系列文章汇总如下,觉得不错点个Star,欢迎 加群 互相学习。
因为篇幅的限制,今天的分享只到这里。如果大家想了解更多的内容的话,可以去扫一扫每篇文章最下面的二维码,然后关注咱们的微信公众号,了解更多的资讯和有价值的内容。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK