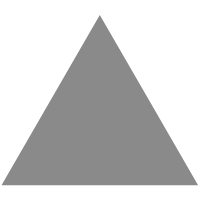
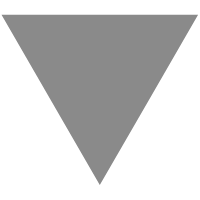
Mybatis精讲(一)---环境配置及架构梳理
source link: https://segmentfault.com/a/1190000020985708
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
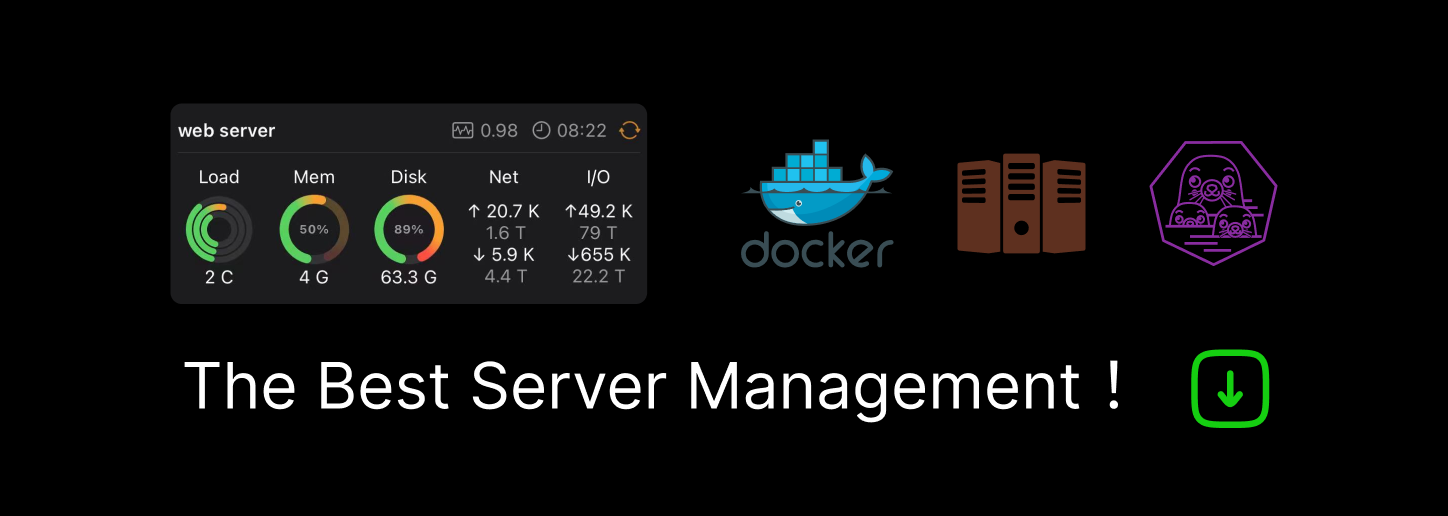
此文已独家授权给【新华前后端开发】使用。其他平台使用联系作者后再使用
[TOC]
简介
在数据库方面我们最常用的应该JDBC、Hibernate和Mybatis。通过JDBC方式连接数据库,我们会发现工作量是相当的复杂。我们得处理一些琐碎的关闭。然后入参出参我们都得自己管理。基于次产生了ORM(Object Relational Mapping)模型。
ORM模型
- 简单的说ORM模型就是数据库表和Java对象的映射模型。主要解决了数据库和Java对象的相互映射。我们可以操作实体对象进而操作数据库表。这样的好处是我们不需要太了解数据库。减轻了我们的学习代价。
Hibernate
- 基于ORM模型很快我们的第二主角登场了。但是由于Hibernate配置比较复杂,且操作性能上不是很好。虽然大大的弱化了我们的sql但是因为性能低下很快就被淘汰了。
Ibatis
- Mybatis的前身严格意义上说应该是Ibatis,后面我们都称之为Mybatis.为了解决Hibernate的不足,Mybatis产生了相对于Hibernate的全表映射Mybatis可以说是半自动映射的框架。因为他是实体和sql结合的一个框架。
- Mybatis有SQL , 实体 , 映射规则三个主要对象主成。和Hibernate相比虽然多出了sql的编写,但是正是因为sql的编写使得Mybatis变得很方便。Hibernate因为不用sql,所以他无法调用存储过程这些数据库方法。但是Mybatis不一样,Mybatis可以调用sql中的存储过程。
环境搭建
jar
<dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis</artifactId> <version>3.5.0</version> </dependency>
配置
- 在上面的图示中我们可以发现,Mybatis中提供的注解或者是接口的方式来操作数据库的。所以这里我们准备一个接口。与接口对应的是xml文件。两者组成了Mybatis中的一个组件。
package com.github.zxhtom.mapper; import com.github.zxhtom.model.Student; import java.util.List; public interface StudentMapper { /** * 获取学生列表 * @return */ public List<Student> getStudents(); /** * 通过id获取学生信息 * @param id * @return */ public Student getStudentByStuId(String id); }
- 与接口对应的是xml,里面记录了真是的sql。这里注意下xml的位置需要和实体在同意文件夹下且同名
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" > <mapper namespace="com.github.zxhtom.mapper.StudentMapper"> <select id="getStudents" resultType="com.github.zxhtom.model.Student"> select * from student </select> <select id="getStudentByStuId" resultType="com.github.zxhtom.model.Student"> select * from student where id=#{id} </select> </mapper>
xml方式配置
- 有了上面的接口和xml,下面我们开始配置我们的Mybatis环境。
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <!--引入外部配置文件--> <properties resource="config.properties"></properties> <!--定义别名--> <typeAliases> <package name=""/> </typeAliases> <!--定义数据库信息,默认使用development数据库构建环境--> <environments default="development"> <environment id="development"> <!--jdbc事物管理--> <transactionManager type="JDBC"></transactionManager> <!--配置数据库连接信息--> <dataSource type="POOLED"> <property name="driver" value="${database.driver}"/> <property name="url" value="${database.url}"/> <property name="username" value="${database.username}"/> <property name="password" value="${database.password}"/> </dataSource> </environment> </environments> <mappers> <mapper resource="com/github/zxhtom/mapper/StudentMapper.xml"></mapper> </mappers> </configuration>
- 然后我们就可以加载这个xml环境配置,构建我们的sqlsession.
//获取mybatis-config.xml位置 InputStream inputStream = Resources.getResourceAsStream(Constant.MYBATIS); //加载mybatis-config,并创建sqlsessionfactory对象 SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream); //创建sqlsession对象 SqlSession sqlSession = sqlSessionFactory.openSession(); Map<String, Object> paramMap = new HashMap<>(); paramMap.put("id", 1); //执行select语句,将resultSet映射成对象并返回 StudentMapper studentMapper = sqlSession.getMapper(StudentMapper.class); List<Student> students = studentMapper.getStudents(); studentPrint(students);
代码方式配置
- 官方提供了另外一种Java对象的方式来配置环境。这种配置我们控制的力度更加细腻,但是导致的问题是我们的一些配置将会硬编码在代码里。我们这里通过Properties文件将硬编码的东西挪出去。
Map<Object, Object> properties = PropertiesUtil.getProperties("config.properties"); PooledDataSource dataSource = new PooledDataSource(); dataSource.setDriver(properties.get("database.driver").toString()); dataSource.setUrl(properties.get("database.url").toString()); dataSource.setUsername(properties.get("database.username").toString()); dataSource.setPassword(properties.get("database.password").toString()); //构建数据库事物方式 TransactionFactory transactionFactory = new JdbcTransactionFactory(); //创建数据库运行环境 Environment environment = new Environment("development",transactionFactory,dataSource); //构建Configure对象 Configuration configuration = new Configuration(environment); //注册别名 configuration.getTypeAliasRegistry().registerAlias("stu", Student.class); //加入一个映射器 configuration.addMapper(StudentMapper.class); //使用sqlsessionfactoryBuilder构建sqlsessionfactory SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(configuration); SqlSession sqlSession = sqlSessionFactory.openSession(); StudentMapper studentMapper = sqlSession.getMapper(StudentMapper.class); List<Student> students = studentMapper.getStudents(); studentPrint(students);
两种方式对比
- 上面我强调了我们的StudentMapper.xml和StudentMapper.java要同名而且在同一位置上。这是为什么呢。因为在Mybatis源码中默认会读取Java对象同目录下的同名的xml文件作为sql配置文件。
- 但是后期我们项目的展开Java和xml会越来越多,放在一起的话很乱。作为一个框架不会这么局限的。在xml配置环境方式中我们是通过Mappers-->Mapper标签来注入我们的Mapper.xml的。这里的xml路径并没有要求在同一个目录。我们这里可以随意的改。上面的那些限制是在Java代码搭建环境的方式中。因为在Java代码方式中我们只是通过java对象注册mapper接口的。在这种方式下默认就会加载同目录下的同名xml
Mybatis结构
- 学习的过程就是认知的过程。接下来我们来认识下Mybnatis的核心组件吧。
- SqlSessionFactoryBuilder : 根据xml(mybatis-config.xml)或者Java代码(代码方式)来生成SqlSessionFactory
- SqlSessionFactory : 构建SqlSession
- SqlSession : 既可以发送sql执行并返回结果,也可以获取Mapper接口
- SQL Mapper : Mybatis独有的组件。有Java接口和xml文件构成的一个整体。负责将xml中的sql进行解析然后传递给数据库并获取结果
源码解读xml环境加载
- 因为范畴基础课程,这里对源码的跟踪点到为止(再深我也不会)。
- 在解析mybatis-config.xml标签这一块是重点。这一块会专门介绍各个标签解析及作用。文章留言或点赞让我们看到你们对这一块的喜爱。留言点赞多的会尽快出这一篇。
映射器解读
- 上面已经说了映射器是有Java+xml组合而成的Mybatis组件。我们上面的案例也展示了两者的编写。Java实际就是一个接口定义好方法。在xml中对应的标签带上我们的sql就行了。
resultMap
Ibatis
- 虽然我们用Mybatis。但是Ibatis之前的方法还是保留下来的。这里提一下。但是不推荐使用的方式执行sql
<span id="addMe">加入战队</span>
微信公众号
Recommend
-
80
Mvvm 前端数据流框架精讲
-
73
-
33
使用了Spring Boot后,能给开发人员带来哪些好处?能给运维人员带来哪些方便?甚至说能给公司带来哪些经济效益?
-
81
本文涉及HashMap的: HashMap的 简单使用 HashMap的 存储结构 原理 HashMap的 扩容方法 原理 HashMap中 定位数...
-
28
开门见山,直接上图,下面的思维导图即是现在要讲的内容,可以先有个印象~ 常见索引类型(实现层面) 索引种类(应用层面) 聚簇索引与非聚簇索引 覆盖索引 最佳索引使用策略 1.常见索引类型(实现层面) 首先不谈Mysql怎么实现索引的,先马后炮一下,如果让
-
34
2019年11月15日阅读 3463精讲 JavaScript 的 "switch" 语句
-
20
博文目录一、RPM包管理工具二、安装、升级和卸载RPM软件三、Linux应用程序基础四、源代码编译安装一、RPM包管理工具RPMPachageManager由RedHat公司提出,被众多Linux发行版所采用。建立统一的数据库文件,详细记录软件包安装、卸载等变化信息,能够自动分析软件包...
-
34
一、什么是 RestTemplate? RestTemplate 是执行HTTP请求的同步阻塞式的客户端,它在HTTP客户端库(例如JDK HttpURLConnection,Apache HttpComponents,okHttp等)基础封装了更加简单易用的模板方法API。也就是...
-
25
本文是精讲RestTemplate第2篇,前篇的blog访问地址如下:
-
23
本文是精讲响应式WebClient第5篇,前篇的blog访问地址如下:
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK