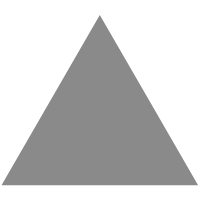
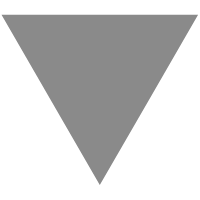
Spring Boot 2.x实战之StateMachine
source link: http://www.javaadu.online/?p=655
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
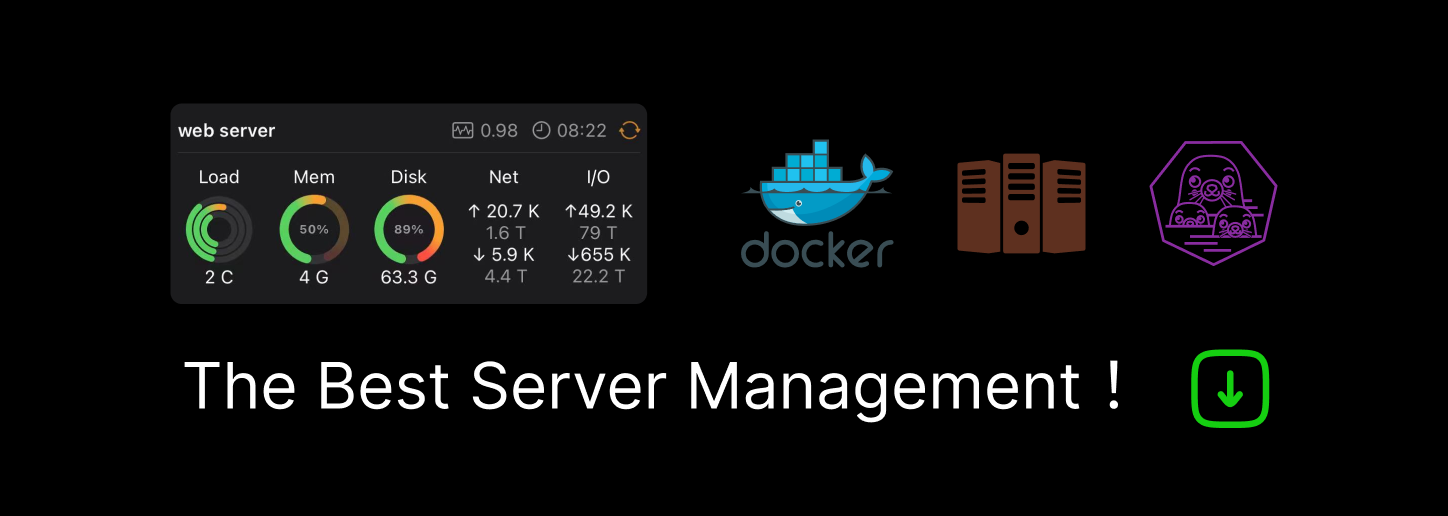
Spring StateMachine是一个状态机框架,在Spring框架项目中,开发者可以通过简单的配置就能获得一个业务状态机,而不需要自己去管理状态机的定义、初始化等过程。今天这篇文章,我们通过一个案例学习下Spring StateMachine框架的用法。
案例介绍
假设在一个业务系统中,有这样一个对象,它有三个状态:草稿、待发布、发布完成,针对这三个状态的业务动作也比较简单,分别是:上线、发布、回滚。该业务状态机如下图所示。
实战
接下来,基于上面的业务状态机进行Spring StateMachine的演示。
- 创建一个基础的Spring Boot工程,在主pom文件中加入Spring StateMachine的依赖:
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.2.1.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <groupId>online.javaadu</groupId> <artifactId>statemachinedemo</artifactId> <version>0.0.1-SNAPSHOT</version> <name>statemachinedemo</name> <description>Demo project for Spring Boot</description> <properties> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <optional>true</optional> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> <exclusions> <exclusion> <groupId>org.junit.vintage</groupId> <artifactId>junit-vintage-engine</artifactId> </exclusion> </exclusions> </dependency> <!--加入spring statemachine的依赖--> <dependency> <groupId>org.springframework.statemachine</groupId> <artifactId>spring-statemachine-core</artifactId> <version>2.1.3.RELEASE</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
定义状态枚举和事件枚举,代码如下:
/** * 状态枚举 **/ public enum States { DRAFT, PUBLISH_TODO, PUBLISH_DONE, } /** * 事件枚举 **/ public enum Events { ONLINE, PUBLISH, ROLLBACK }
- 完成状态机的配置,包括:(1)状态机的初始状态和所有状态;(2)状态之间的转移规则
@Configuration @EnableStateMachine public class StateMachineConfig extends EnumStateMachineConfigurerAdapter<States, Events> { @Override public void configure(StateMachineStateConfigurer<States, Events> states) throws Exception { states.withStates().initial(States.DRAFT).states(EnumSet.allOf(States.class)); } @Override public void configure(StateMachineTransitionConfigurer<States, Events> transitions) throws Exception { transitions.withExternal() .source(States.DRAFT).target(States.PUBLISH_TODO) .event(Events.ONLINE) .and() .withExternal() .source(States.PUBLISH_TODO).target(States.PUBLISH_DONE) .event(Events.PUBLISH) .and() .withExternal() .source(States.PUBLISH_DONE).target(States.DRAFT) .event(Events.ROLLBACK); } }
- 定义一个测试业务对象,状态机的状态转移都会反映到该业务对象的状态变更上
@WithStateMachine @Data @Slf4j public class BizBean { /** * @see States */ private String status = States.DRAFT.name(); @OnTransition(target = "PUBLISH_TODO") public void online() { log.info("操作上线,待发布. target status:{}", States.PUBLISH_TODO.name()); setStatus(States.PUBLISH_TODO.name()); } @OnTransition(target = "PUBLISH_DONE") public void publish() { log.info("操作发布,发布完成. target status:{}", States.PUBLISH_DONE.name()); setStatus(States.PUBLISH_DONE.name()); } @OnTransition(target = "DRAFT") public void rollback() { log.info("操作回滚,回到草稿状态. target status:{}", States.DRAFT.name()); setStatus(States.DRAFT.name()); } }
- 编写测试用例,这里我们使用CommandLineRunner接口代替,定义了一个StartupRunner,在该类的run方法中启动状态机、发送不同的事件,通过日志验证状态机的流转过程。
public class StartupRunner implements CommandLineRunner { @Resource StateMachine<States, Events> stateMachine; @Override public void run(String... args) throws Exception { stateMachine.start(); stateMachine.sendEvent(Events.ONLINE); stateMachine.sendEvent(Events.PUBLISH); stateMachine.sendEvent(Events.ROLLBACK); } }
在运行上述程序后,我们可以在控制台中获得如下输出,我们执行了三个操作:上线、发布、回滚,在下图中也确实看到了对应的日志。不过我还发现有一个意料之外的地方——在启动状态机的时候,还打印出了一个日志——“操作回滚,回到草稿状态. target status:DRAFT”,这里应该是状态机设置初始状态的时候触发的。
分析
如上面的实战过程所示,使用Spring StateMachine的步骤如下:
- 定义状态枚举和事件枚举
- 定义状态机的初始状态和所有状态
- 定义状态之间的转移规则
- 在业务对象中使用状态机,编写响应状态变化的监听器方法
为了将状态变更的操作都统一管理起来,我们会考虑在项目中引入状态机,这样其他的业务模块就和状态转移模块隔离开来了,其他业务模块也不会纠结于当前的状态是什么,应该做什么操作。在应用状态机实现业务需求时,关键是业务状态的分析,只要状态机设计得没问题,具体的实现可以选择用Spring StateMachine,也可以自己去实现一个状态机。
使用Spring StateMachine的好处在于自己无需关心状态机的实现细节,只需要关心业务有什么状态、它们之间的转移规则是什么、每个状态转移后真正要进行的业务操作。
本文完整实例参见: https://github.com/duqicauc/Spring-Boot-2.x-In-Action/tree/master/statemachinedemo
参考资料
- http://blog.didispace.com/spring-statemachine/
- https://projects.spring.io/spring-statemachine/#quick-start
本号专注于后端技术、JVM问题排查和优化、Java面试题、个人成长和自我管理等主题,为读者提供一线开发者的工作和成长经验,期待你能在这里有所收获。

Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK