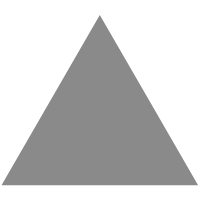
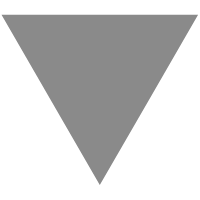
Node.js library for controlling Xiao Mi Yeelight
source link: https://www.tuicool.com/articles/7NNBRza
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
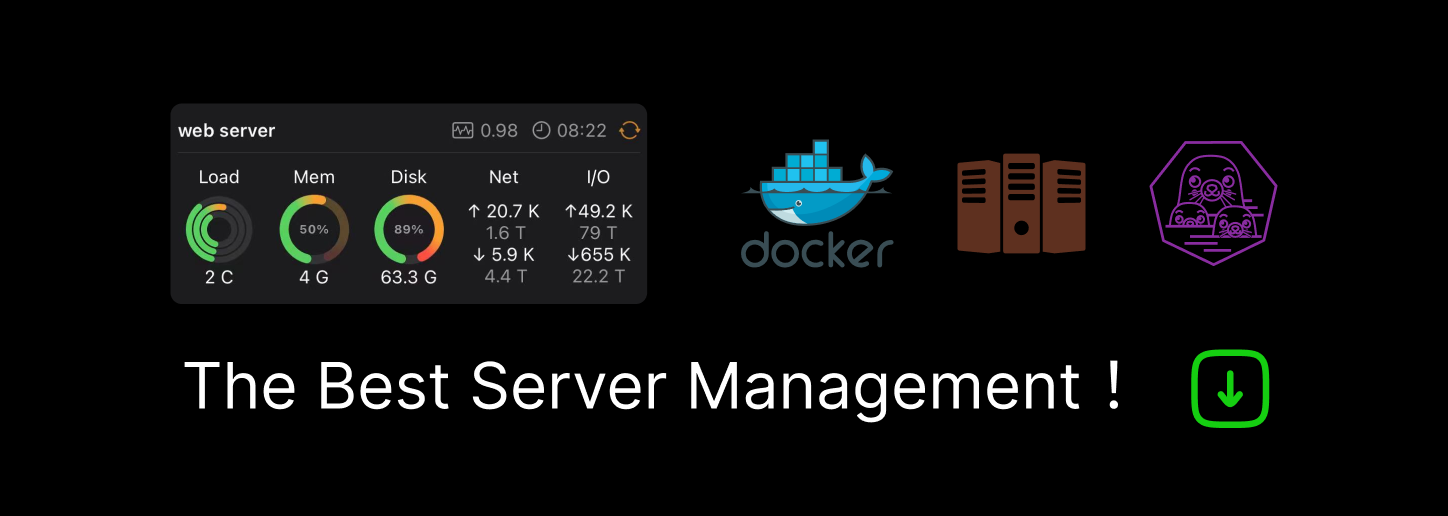
yeelight.io
Description
yeelight.io
is a simple library for you to control YeeLight LED bulb through LAN.
Installation
npm install yeelight.io
Usage
Using Bulb type
'use strict'; const { Bulb } = require('yeelight.io'); const l1 = new Bulb('192.168.1.227'); l1.on('connected', (light) => { console.log(`connected to ${light.ip}`); light.toggle(); light.disconnect(); }); l1.on('disconnected', (light) => { console.log(`disconnected with ${light.ip}`); }); l1.on('error', (light, err) => { console.error(`error [${err.message}] occur on ${light.ip}`); light.disconnect(); }); l1.connect();
Using pre-implement methods
'use strict'; const { toggle } = require('yeelight.io'); toggle('192.168.1.227', (err) => { if (err) { console.error(`error [${err.message}] occured on 192.168.10.227`); } else { console.log('toggle 192.168.1.227 success'); } });
API
-
Bulb(ip, [port])
-
on(ip, [cb(err)])
-
off(ip, [cb(err)])
-
brightness(ip, [cb(err)])
-
color(ip, [cb(err)]
Bulb(ip, [port])
Create a new Bulb object
Methods:
Events:
-
Event: 'connected'
-
Event: 'disconnected'
-
Event: 'error'
instance.connect()
Start connecting to Yeelight bulb
const l1 = new Bulb(IP_STR); l1.connect();
Instance.toggle()
Toggle a Yeelight bulb
const l1 = new Bulb(IP_STR); ... l1.toggle();
Instance.onn()
Turn on a Yeelight bulb. NOTE
: onn
on purpose to avoid same name with event on
const l1 = new Bulb(IP_STR); ... l1.onn();
Instance.off()
Turn off a Yeelight bulb
const l1 = new Bulb(IP_STR); ... l1.off();
Instance.brightness(level)
Change brightness of a Yeelight bulb
const l1 = new Bulb(IP_STR); ... l1.brightness(50); // Turn brightness to half
Instance.color(r, g, b)
Change color of a Yeelight bulb
const l1 = new Bulb(IP_STR); ... l1.color(255, 0, 0); // Turn bulb to red
Event: 'connected'
Emit when connected with Yeelight bulb
-
light
<Bulb>
bulb that is connected
Event: 'disconnected'
Emit when disconnected with Yeelight bulb
-
light
<Bulb>
bulb that is disconnected
Event: 'error'
Emit when any kind of error occured
-
light
<Bulb>
-
err
<Error>
Event: 'data'
Emit when Yeelight bulb sends response
-
light
<Bulb>
-
data
<object>
toggle(ip, [cb(err)])
Toggle a Yeelight bulb
-
ip
<string>
eelight bulb IP address -
cb(err)
<Function>
called after toggle command is sent to the bulb,err
<Error>
not null if error occured
on(ip, [cb(err)])
Turn on a Yeelight bulb
-
ip
<string>
eelight bulb IP address -
cb(err)
<Function>
called after toggle command is sent to the bulb,err
<Error>
not null if error occured
off(ip, [cb(err)])
Turn off a Yeelight bulb
-
ip
<string>
eelight bulb IP address -
cb(err)
<Function>
called after toggle command is sent to the bulb,err
<Error>
not null if error occured
brightness(ip, level, [cb(err)])
Change brightness of a Yeelight bulb
-
ip
<string>
eelight bulb IP address -
level
<number>
brightness level, 0 ~ 255 -
cb(err)
<Function>
called after toggle command is sent to the bulb,err
<Error>
not null if error occured
color(ip, r, g, b[cb(err)])
Change color of a Yeelight bulb
-
ip
<string>
eelight bulb IP address -
r
<number>
red of RGB, 0 ~ 255 -
g
<number>
green of RGB, 0 ~ 255 -
b
<number>
blue of RGB, 0 ~ 255 -
cb(err)
<Function>
called after toggle command is sent to the bulb,err
<Error>
not null if error occured
License
MIT
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK