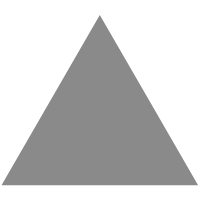
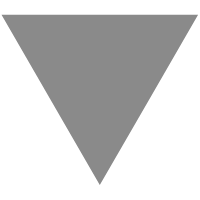
A definitive guide to Redux vs. MobX
source link: https://www.tuicool.com/articles/eYzQ7rB
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
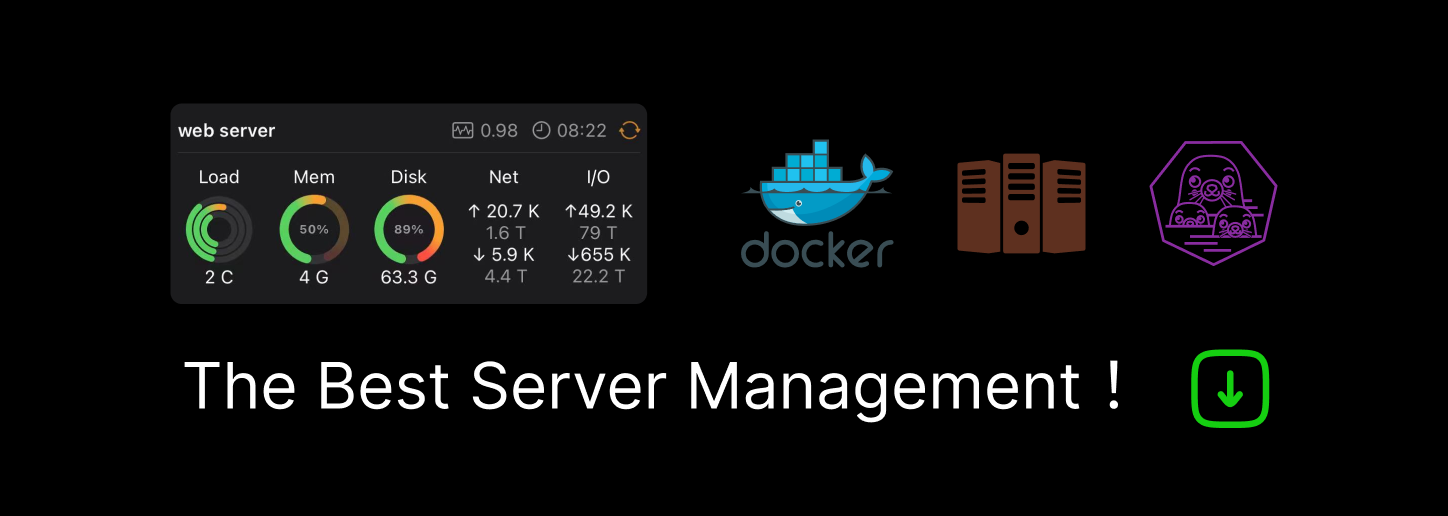
One of the hardest problems to solve in large front-end applications is state management. While there are several approaches to solving state management problems, Redux and MobX are two of the most popular external libraries used to address state management in front-end applications. In this post, we will look at each library and how they match up.
This article assumes that you have a basic idea of how state management works within your web application. Both Redux and MobX are framework agnostic and will work with any framework or plain vanilla JavaScript.
Redux
Redux is a popular state management solution that is a combination of both Flux and functional programming concepts. Some of the core principles of Redux are:
- Redux has a single store – a single source of truth
- The state in the store is immutable
- Actions invoke changes to the store
- Reducers update state
MobX
MobX is a state management solution that helps in managing the local state within your app.
Some of the core principles of MobX are:
- MobX can have multiple stores to store the state of the application
- Anything that can be derived from the state without any further interaction is a derivation
- Action is any piece of code that can change the state
- All d erivations are updated automatically and atomically when the state change
Now let’s compare some of the key features of Redux vs. MobX to see what suits your needs better.

Popularity
Before beginning your quest to learn Redux or MobX, let’s look at which one is more popular.
Take a look at the Google Trends graph below. As of April 2019, Redux appears to be a much more popular and searched concept on Google, in comparison to MobX.
To get more insight into their popularity factors, let’s take a look at the State of JavaScript survey of 2018 . It released data on both Redux and MobX popularity over the last three years among developers.
Redux
MobX
Over the last few years, Redux has gained a ton of popularity and has been the go – to solution for state management. Although MobX is not as popular as Redux, it has its own perks as well.
Winner: Redux
Learning curve
Redux
The popular opinion that developers have about Redux is that it is not easy to learn. The State of JavaScript survey of 2018, analy z ed the most disliked aspects of redux . Here, developers have voted that they don’t like the complex nature of Redux and the hard learning curve that it comes with.
It takes some time to understand its patterns and paradigms. It is a combination of the Flux architecture and functional programming concepts. If you are a functional programmer, you may find it easier to grasp Redux. Whereas if you come from an object-oriented programming background, Redux code looks complex and hard to understand initially.
Learning Redux, also means you need to learn about Redux middleware like Thunk s or Sagas , which adds more material to learn.
MobX
MobX is known to be much easier to grasp when compared to Redux. Most JavaScript developers are well versed in o bject -o riented p rogramming, which makes learning MobX simple. Also, there are a lot of things that are done behind the scenes in MobX, creating a better learning experience for the developers. You wouldn’t need to worry about normalizing the state or implement concepts like Thunks. It leads to writing less code since the abstraction is already built-in.
Winner: MobX
Storing data – single store vs. multiple stores
The store is where we will store the local data. It holds the entire application’s state. The store typically holds the application’s state in a huge JSON object.
Redux
In Redux, there is only one store and it is the single source of truth. The state in the store is immutable , which makes it easier for us to know where to find the data/state. In Redux, although there is one giant JSON object that represents the store, you can always split the code into multiple reducers. This way you can logically separate the concerns with multiple reducers. This is a more intuitive approach for many developers since they can always refer to the single store for the application’s state and there is no possibility of duplication or confusion related to the current state of the data.
MobX
MobX, on the other, hand allows multiple stores. You can logically separate stores , so all of the application’s state is not in one store . Most applications are designed to have at least two stores. One for the UI state and one or more for the domain state . The advantage of separating the stores this way is that you can re-use the domain in other applications as well. And the UI store would be specific to the current application.
Winner: Redux
Data structure
Redux
<
MobX
MobX uses observable data. This helps in automatically tracking changes through implicit subscriptions. In MobX, the updates are tracked automatically. Therefore, making it easier for the developer.
Winner: MobX
Pure vs. i mpure
Redux
In Redux the state in the store is immutable, which means all of the states are read-only. Actions in Redux can invoke changes to state and the reducers can replace the previous state with a new state. This is one of the core principles of Redux.
A simple example of a pure function is shown below:
function sumOfNumbers(a, b) { return a + b; }
The function will always return the same output, given the same input. It does not have any side effects or influence from the outside world.
Redux functions are written with the following pattern. Reducers are pure functions that take in a state and action and return a new state.
function(state, action) => newState
This makes Redux pure. If you are interested in learning more about pure functions and how they operate in Redux, you can read this article for a better understanding. This i s one of the best features of Redux.
MobX
In MobX the state is mutable, which means you can simply update the state with new values. This makes MobX impure. Impure functions are harder to test and maintain since they don’t always return predictable outputs.
Winner: Redux
Since the Redux store is pure, it is more predictable and it is easy to revert state updates. In the case of MobX, if not done right, the state updates could make it harder to debug.
Boilerplate code
Redux
One of the biggest complaints about Redux is the amount of boilerplate code that it comes with. And when you integrate React with Redux, that results in even more boilerplate code. This is because Redux is explicit in nature and a lot of the capabilities have to be explicitly coded.
MobX
MobX is more implicit and does not require a lot of special tooling. It comes with much less boilerplate code in comparison to Redux. This makes MobX, easier to learn and setup.
Winner: MobX
Develop er c ommunity
In regard to the developer community, Redux wins hands down. Redux comes with the “Redux Dev Tools” that is used by thousands of developers. It offers amazing support for debugging Redux code.
MobX also offers developer tools, but they do not have the same quality of debugging support that Redux provides.
The GitHub stats Redux has about 48k stars, with over 672 contributors. MobX , on the other hand , has around 19k stars and 155 contributors.
If we look into the downloads from npm, Redux is way ahead. Redux averages 3 Million downloads a week and MobX averages about 254k downloads a week. This shows how widely Redux has been adopted.
Winner: Redux
Scalability
Since Redux is more opinionated and expects the reducer functions to be pure , it is easier to scale than MobX. The opinionated and pure nature of Redux enables scalability of the apps.
Pure functions are easier to test since they are predictable and simple. This results in maintainable code that can scale. This is one of the core benefits of choosing Redux over MobX.
Winner: Redux
Conclusion
Alright, what’s the verdict? Based on the developer community, popularity and scalability Redux performs better than MobX. But if you were looking to build simple apps, with less boilerplate code that you want to learn quickly, MobX might be your friend.
Plug:LogRocket, a DVR for web apps
LogRocketis a frontend logging tool that lets you replay problems as if they happened in your own browser. Instead of guessing why errors happen, or asking users for screenshots and log dumps, LogRocket lets you replay the session to quickly understand what went wrong. It works perfectly with any app, regardless of framework, and has plugins to log additional context from Redux, Vuex, and @ngrx/store.
In addition to logging Redux actions and state, LogRocket records console logs, JavaScript errors, stacktraces, network requests/responses with headers + bodies, browser metadata, and custom logs. It also instruments the DOM to record the HTML and CSS on the page, recreating pixel-perfect videos of even the most complex single-page apps.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK