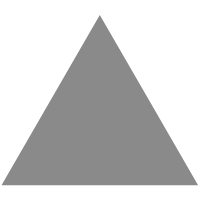
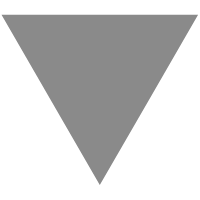
Create Adobe XD plugin using JavaScript, HTML, and CSS
source link: https://www.tuicool.com/articles/hit/MziMzaJ
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
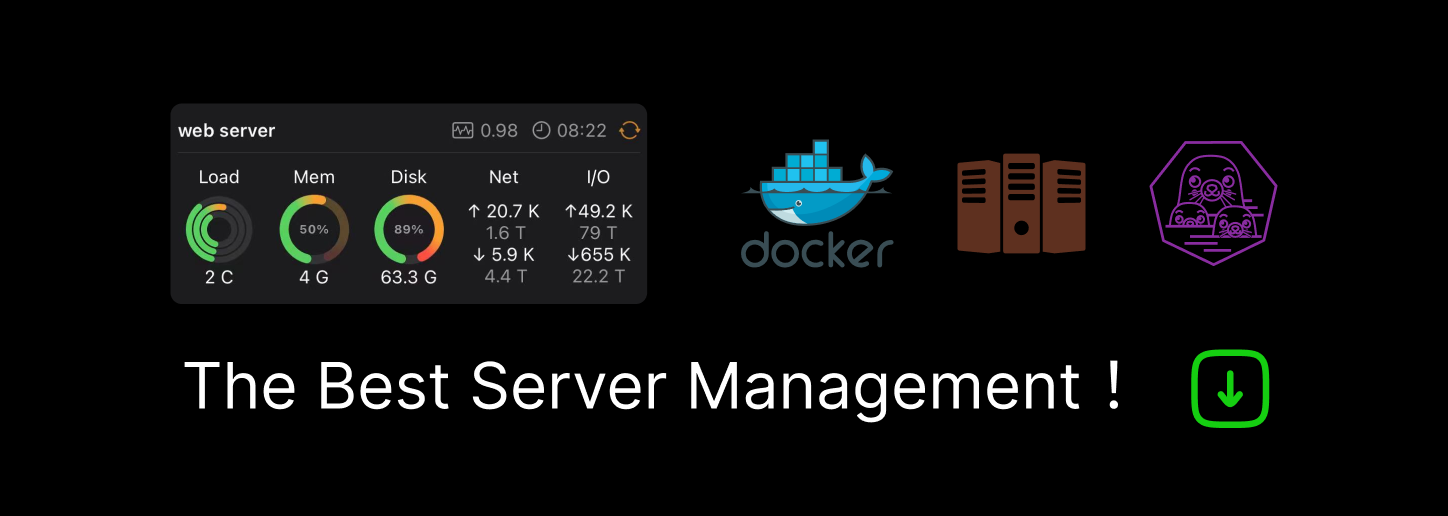
Spinning Up Your First Adobe XD Plugin
So, you might have heard that Adobe XD now supports plugins and are curious how to get started building your own. Well, great news — building a plugin is super easy. In this post, I’ll take you through the process, step-by-step.
Have you heard? We’re doing an Adobe XD plugin workshop in London (February 25), Amsterdam (February 27), and Mannheim (March 1). Visit http://bit.ly/helloxdplugin for more details! We’d love to see you there!
Install/update Adobe XD
The first thing you should do is ensure that you’ve got the most recent version of Adobe XD installed on your computer. If you’ve previously installed XD, open the Adobe Creative Cloud app and click the “Update” button next to the XD app.

If you haven’t previously installed XD, the button above will be labeled “Install” instead — just click it and XD will install on your machine.
Get a feel for what’s possible
XD has launched with several amazing plugins ranging from utility plugins that simplify repetitive tasks to plugins that integrate with cloud storage and other services. To get a feel for what plugins can do, you should definitely try a few of these out.
- Launch XD
- Create a new document
- Open the Plugins menu, and then click Discover Plugins .


Installing a plugin is as easy as clicking the “Install” button. You don’t even have to restart XD to start using them.
Plan your plugin
Now that you know what’s possible, it’s time to think about what kind of plugin you want to create. Let’s riff on the version in the Quick Start documentation and put our own custom spin on that plugin. But first, some context!
Let’s imagine that you’re a designer building out a banner for a conference. This conference happens to be all about spirals in design. The conference itself is named “ConSpiralator ’19.”


You’ve got a basic banner, but your manager insists that it should be more visually compelling. “Maybe something with spirals,” she says.


Ok — that’s not hard to do with a lot of rectangles or other shapes, but it could also be really tedious to build. A stock image might work, but you’re going to want this to scale and fit within the design. And chances are good your manager is going to want to change aspects of the design after you’re done.
Plugins to the rescue! Instead of taking hours painstakingly making spirals by hand, you could have a plugin do it for you. So, let’s do that!
Open the plugin development folder
XD has a “development mode” that makes it really easy to start building and testing your plugin. This happens to be a folder named “develop” that XD creates for you. Any plugins within this folder can be reloaded using a keyboard shortcut, which allows for rapid iteration while playing with your plugin. Best of all, you don’t have to restart XD every time you want to test a new version of your plugin!
XD makes it really easy to open this folder without having to drill down into the file system using Finder or Explorer. All you need to do is use the Plugins > Development > Show Develop Folder menu command, and a new Finder or Explorer window will open, revealing the “develop” folder.




Create your plugin folder
Because you can have many plugins under development at once, you don’t build your plugin directly in the “develop” folder. Instead, XD expects that you will create a folder for each plugin you want to build. The name of the folder itself isn’t important, but it should be something you’ll recognize.
Let’s create a folder for our first plugin. We can name it “my-first-plugin” or just about anything you want (just be sure to name it something you’ll recognize).




Create your plugin’s manifest
All XD plugins are required to have a “manifest.” A manifest is just a list of facts about your plugin, including things like the name of the plugin, and the menu item you want to use. Manifests are really easy to build, and generally you’ll find yourself copying and pasting from ones you’ve created before. In fact, you can use the one below:
{ "name": "Draw Lots of Rectangles", "id": "00000000", "version":"1.0.0", "description":"Sample plugin for Adobe XD...", "icons":[ { "width":96, "height":96, "path":"images/icon.png" } ], "host":{ "app":"XD", "minVersion":"13.0.0" }, "uiEntryPoints":[ { "type":"menu", "label":"Draw lots of rects", "commandId":"myPluginCommand" } ] }
When you save this, make sure the file is named manifest.json
or your plugin won’t load correctly.
There are two main things I want to point out here:
- The label inside uiEntryPoints is what will be displayed to the user in the Plugins menu.
- The commandId tells XD what function to call when the user invokes our menu item.
For more information on what each part does, please see the plugin structure documentation .
Create your plugin’s code
XD Plugins need at least one more file in order to function: the plugin logic or code. This resides in a file named main.js
. (Note that you aren’t limited to only this file, but all plugins must have at least one file with this name.)
Your plugin’s code needs to do the following:
- Declare what APIs your plugin needs to use. In the case of XD, this might be an indication that your plugin needs to draw rectangles or other shapes.
- Implement your plugin’s menu handlers. Right now your plugin can only be invoked via the Plugins menu. XD needs to know how to “handle” your menu items when they are invoked.
- Export your menu handlers to XD. Think of this as wiring up your menu handlers to the command IDs used in the manifest.
Typically you’ll start with a scaffold that lets you test each step as you go. So let’s build that out first.
const { Rectangle, Color } = require("scenegraph"); // [1] const commands = require("commands"); // [2]
function myPluginCommand(selection) { // [3] /* all the code we talk about in the next section will go in here */ }
module.exports = { // [4] commands: { myPluginCommand } };
Let’s start from the top, shall we?
First, at the top of the file, we need to declare what APIs we need. That is, we’re going to need certain modules from XD. That’s easy to to do in a couple lines of code ([1] and [2]). In the first line, we’re asking for the ability to create rectangles and use colors — easy enough, right? There are other specific types of shapes and classes you can ask for as well, and those are all available in our comprehensive documentation .
[2] asks for the ability to perform certain kinds of commands on the XD document — in our case, we need to be able to duplicate shapes, but there are other commands such as the ability to send shapes to the background or foreground, group shapes, and more.
[3] is the function we’ll use to contain our plugin logic. To make it easy, we’ll name it myPluginCommand
, which is the same as in manifest.json
. We’ll finish filling this out in a second.
Note:This function is called a menu handler because it’s what will run when the user invokes our plugin from the Plugins menu.
[4] exports the menu handler to XD. Note that we reuse myPluginCommand
from the manifest.json
. This must match the manifest, or your plugin won’t work correctly.
Cool — with the boilerplate out of the way, we can start writing our code. Be sure to put everything inside the myPluginCommand
function.
One more thing: one of the coolest things about developing plugins with XD is that you don’t even need to quit and relaunch XD whenever you change your plugin code. You just need to let XD know that it should load your plugin again. This is done with the Plugins > Development > Reload Plugins menu item. There’s a corresponding keyboard shortcut ( Cmd + Shift + R on macOS and Ctrl + Shift + R on Windows) for even faster reloading.
Once you reload the plugins, you should be able to see your plugin in the Plugins menu. If you don’t see it, check the developer console for any error messages ( Plugins > Development > Developer Console ).
Now, at the end of each step below, you should be able to reload the plugin and click Plugins > Draw lots of rects to see your latest changes.
Note:You’ll probably want to create a new document in XD and change the artboard’s background to a dark color (like black or blue). We’ll be working with white objects, so they’ll be hard to see otherwise.
Implement your plugin logic
This is where the real work happens; we have to define our plugin’s logic. Thankfully, when automating a tedious workflow, the steps are usually fairly obvious. Let’s go over each one.
Create a rectangle
Creating shapes in XD is really easy — all we have to do is ask for a new shape and provide some basic information (such as the size).
const rect = new Rectangle(); rect.width = 320; rect.height = 320;
Of course, what we’re really creating here is a square (since the width and height are equal).
Change the rectangle style
By default, rectangles are created with a white fill color. In our case, we don’t want the rectangle to be filled at all. Instead we want a white stroke. That’s easy to do too!
rect.fill = null; rect.stroke = new Color("white"); rect.opacity = 0.5;
In line 1, we’ve set the fill to null — this ensures that the solid white fill is removed. Then in line 2, we ask for a color ( white
). This color can be a named CSS color, a hex color (like #FFFFFF
), or even an RGB/RGBA or HSL/HSLA value.
Add the rectangle to the canvas
If we stopped here, we’d not actually see anything on our canvas. We have to add it to the document itself.
selection.insertionParent.addChild(rect);
All the above line does is add the rectangle next to whatever the user had selected when they invoked the plugin.
Select the rectangle
Next, we want to select the new rectangle, just as if we had clicked it in the XD interface with our mouse.
selection.items = [rect];
Duplicate the selection
When you’re performing a copy & paste operation, you first select whatever it is you want to duplicate. The same is true when duplicating objects in a plugin, which is why we first selected the rectangle in the previous step. Now let’s duplicate that selection:
commands.duplicate();
Hmm — this wouldn’t actually look all that different on screen. commands.duplicate
will copy everything about the shape, including its position. That means we’ve created a rectangle directly on top of the previous rectangle!
Rotate it
If we rotated the rectangle, we’d see two distinct rectangles clearly. That’s easy!
const node = selection.items[0];node.rotateAround(5, node.localCenterPoint);
The first thing we do (line 1) is get the first item currently selected. node will refer to the duplicated rectangle. Then we rotate it (line 2) by 5
degrees around the rectangle’s center. It’s worth trying various values until you get something you like.
Note:the only reason we assigned the first selected item to node
is because we’re going to repeat that a few times over. It’s easier to type node
than it is to type selection.items[0]
each time!
Move it
If we rotated a square a lot of times in place, it’d give us an awesome result, but it wouldn’t be wide enough to fill our banner. Let’s move the duplicate to the right a little instead.
node.moveInParentCoordinates(5, 0);
Ah — that works! This moves the new rectangle five pixels to the right. The first number is the horizontal movement, and the second is the vertical movement. The final result depends in part on what two numbers you use here! Play around — experiment!
Repeat!
Great — we’ve built two rectangles. That’s not a spiral. We need to repeat all of this. We can do that with a for loop or a while loop. (Any looping mechanism will do!)
So let’s take steps 6–9 and add a loop:
let times = 0; while (times < 179) {
// steps 6 - 9 commands.duplicate(); const node = selection.items[0]; node.rotateAround(5, node.localCenterPoint); node.moveInParentCoordinates(5, 0); // end of steps 6 - 9 times += 1; }
Now, when you run your plugin on an artboard with a dark color (because we’re building white rectangles), you’ll see this:


Wow, isn’t that cool? Pretty amazing for 32 lines of code, right?
Where to go from here
Excited yet? Plugins can do some pretty amazing things, and they aren’t hard to write, either. Definitely check out the following resources for more useful information, samples, and guides.
- Your hub for everything related to XD Plugins
- Documentation
- Quick Start
- API Reference
- Plugin Samples (This sample is there too !)
- Community Resources
We can’t wait to see what you’ll build!
Follow the Adobe Tech Blog for more developer stories and resources, and check out Adobe I/O on Twitter for the latest news and developer products.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK