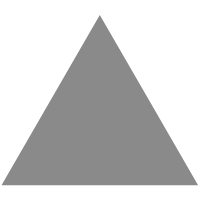
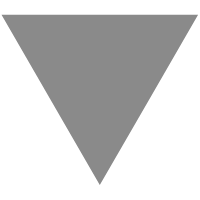
Go slice(四)
source link: https://studygolang.com/articles/18770?amp%3Butm_medium=referral
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
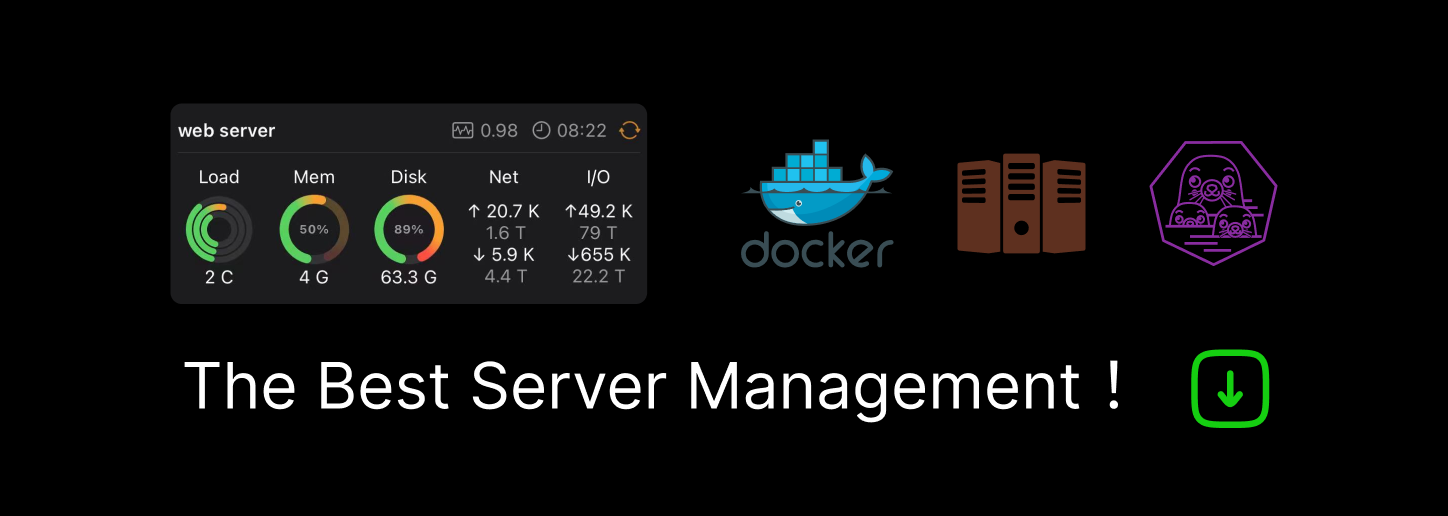
Golang的数据声明格式如下
data := [7]int{}
data:=[3]int{1, 2, 3}
data := [...]int{1, 2, 3}
我们注意到,与其他语言不同的是数组的大小放在前面。
Go 语言的数组当做参数时,是值传递,即会copy数组中的所有数据。
所以在实际的go语言开发中其实很少使用数组,而是使用slice.
所以下面我们重点介绍一下slice
data := []int{}
data := []int{1,2, 3}
slice的声明和array类似,只是省略了大小或者'...'
当slice的空间不足时,它就会自动将存储空间增加一倍。
可以用len(data)获取slice中数据的长度,用cap(data)获取slice的容量(capacity).
func printSlice(data []int){ for _, ele := range data{ fmt.Print(ele, " ") } fmt.Println() } func main(){ data := []int{1,2,3,4} printSlice(data) fmt.Printf("Capacity: %d, Length: %d\n", cap(data), len(data)) data = append(data, 10) printSlice(data) fmt.Printf("Capacity: %d, Length: %d\n", cap(data), len(data)) } ------------------------------------------ //result 1 2 3 4 Capacity: 4, Length: 4 1 2 3 4 10 Capacity: 8, Length: 5
上述代码生声明一个slice, 初始容量和数据长度都是4,然后通过append在slice后面动态添加了一个数据10, 此时由于容量已经等于已有数据长度,即slice已满,所以需要动态扩容, go扩容方案是直接在原来的基础上加倍 , 所以我们看到长度增加了1,但是容量增加了4
所以这里需要注意的就是,如果slice容量已经很多,且满了。那么此时添加一条记录的代价将是非常高的。
我们也可以通过make函数来生成slice. 通过make函数还可以指定slice的初始大小
data := make([]byte, 5)
*说到make,这里我们提一下go语言的内存分配, go 有两个内建函数来分配内存,new 和make. 两者的区别是,new只是分配存储空间,make则主要用于slice, map, channel的初始化
copy() 函数
copy(dst, src): copy函数将src slice的数据拷贝到dst slice里面
其中,
- dst, src必须是slice, 如果是array,则必须通过array[0:] 转换成slice
-
copy时,src数据会覆盖dst里面原来的数据, 如果src数据量小于dst数据量,那么dst里面会有一部分数据不被覆盖;如果src数据量大于dst,那么将只copy dst.length长度的src数据
我们通过下面的实例来体会一下
package main import "fmt" func main(){ a6 := []int{1,2,3,4,5,6} a4 := []int{-1,-2,-3,-4} fmt.Println("a6:", a6) fmt.Println("a4:", a4) copy(a6, a4) fmt.Println("copy a4 to a6, ", a6) fmt.Println() a6 = []int{1,2,3,4,5,6} a4 = []int{-1,-2,-3,-4} copy(a4, a6) fmt.Println("copy a6 to a4, ", a4) fmt.Println() array5 := [5]int{5,-5,5,-5,5} s7 := []int{7,7,7,7,7,7,7} fmt.Println("array5 ", array5) fmt.Println("s7 ", s7) copy(array5[0:], s7) fmt.Println("copy s7 to array5 ", array5) } -------------------------------------------- //result a4: [-1 -2 -3 -4] copy a4 to a6, [-1 -2 -3 -4 5 6] copy a6 to a4, [1 2 3 4] array5 [5 -5 5 -5 5] s7 [7 7 7 7 7 7 7] copy s7 to array5 [7 7 7 7 7]
Recommend
-
85
GitHub is where people build software. More than 27 million people use GitHub to discover, fork, and contribute to over 80 million projects.
-
63
本文翻译自 SliceTricks 。我会追加一些我的理解。官方给出的例子代码很漂亮,建议大家多看看,尤其是利用多个切片共享底层数组的功能就地操作很有意思。 ...
-
37
The hard work isn’t over just because your app has racked up a ton of downloads and positive reviews on the Google Play store. Your typical mobile user has dozens of apps installed on their device, and with new...
-
57
slice 和 array 要说 slice,那实在是太让人熟悉了,从功能上讲 slice 支持追加,按索引引用,按索引范围生成新的 slice,自动扩容等,和 C++ 或 Java 中的 Vector 有些类似,但也有一些区别。 不过 Go 里的 slice 还...
-
58
Slice of PIE is a new mini-series
-
41
Go中的Array和Slice 翻译来于: https://blog.golang.org/slices 操作 extend func Extend(slice []int, element int) []int { n...
-
91
翻Golang代码: type slice struct { array unsafe.Pointer len int cap int } slice 的array是一个指针,指向一块连续内存。 再看 growslice 函数,这是append调用的函...
-
48
本文基于golang 1.10版本分析。 slice 结构 slice实际就是一个struct,在runtime/slice.go中的定义如下: type slice struct { array unsafe.Pointer len int cap int } // An notInHe...
-
38
1、简介 Go的 Slice(切片) 类型提供了一种方便有效的方法来处理类型化数据序列。 slice类似于其他语言中的数组,但具有一些不寻常的属性。 本文将介绍切片是什么以及如何使用它们。 2、Slices
-
43
原文地址:
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK