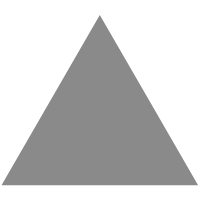
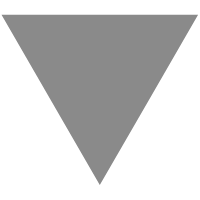
Pull to Refresh iOS Tutorial
source link: https://www.tuicool.com/articles/hit/Efq6Fry
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
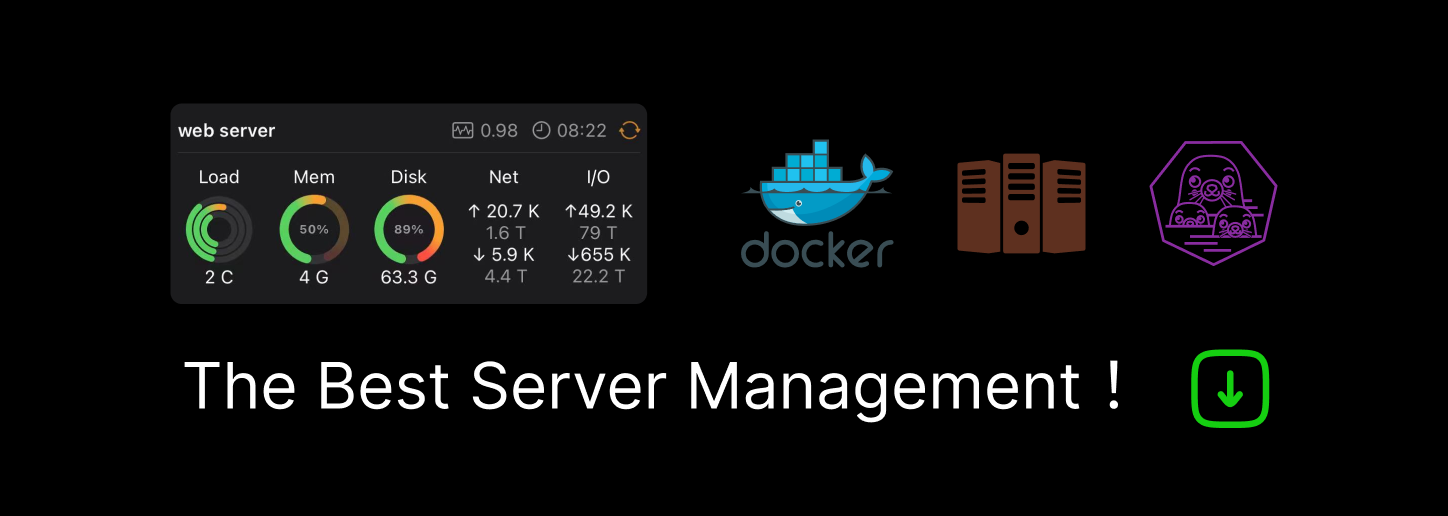
A UIRefreshControl object provides a standard control that can be used to initiate the refreshing of a table view’s contents. When pulled, a little wheel starts spinning at the top, until the refresh has completed. At that time, the wheel disappears, and the view bounces back into place. In this tutorial a refresh control will be added to a table view. When the control is pulled the sorting order of the rows will be reversed. This tutorial is made with Xcode 10 and built for iOS 12.
Open Xcode and create a new Single View App.

For product name, use IOSPullToRefreshTutorial and then fill out the Organization Name and Organization Identifier with your customary values. Enter Swift as Language and choose Next.

Go to the storyboard . Remove the View Controller from the Storyboard and drag a Navigation Controller to the Scene. This will also add the Table View Controller . Select the Navigation Controller and go to The Attribute inspector. In the View Controller section check the "Is Initial View Controller" checkbox.

Select the Table View Cell and go to the Attributes Inspector. In the Table View Cell section set the Identifier to " Cell" .

The storyboard will look like this.

Since the View Controller is removed from the Storyboard the ViewController.swift file can also be deleted from the project. Add a new file to the project, select iOS->Source->Cocoa Touch Class. Name it TableViewController and make it a subclass of UITableViewController.

The TableViewController class needs to be linked to The Table View Controller object in the Storyboard. Select it and go the Identity Inspector. In the Custom Class section change the class to TableViewController.

Go to TableViewController.swift and create a property containing an array of the first letters of the alphabet.
var alphabet = ["A","B","C","D","E","F","G","H","I"]
The TableViewController class contains some boilerplate code. Change the following delegate methods
override func numberOfSections(in tableView: UITableView) -> Int { // 1 return 1 } override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int { // 2 return alphabet.count } override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell { // 3 let cell = tableView.dequeueReusableCell(withIdentifier: "Cell", for: indexPath) cell.textLabel?.text = alphabet[indexPath.row] return cell }
-
There is only one section in the Table View so 1 needs to be returned in the numberOfSections(in:) method.
-
The number of rows is equal to the number of items in the array so the count property of the array class is used.
-
The letter of the alphabet at the current index of the apps array is assigned to the text property of the textLabel property of the current cell.
Build and Runthe project.

Now that some rows are filled let's get going with the refresh control. If the pull to refresh is initiated the rows sort in descending/ascending order. First a Boolean is needed to switch the sort order. Add the following property in the interface section.
var isAscending = true
Change the viewDidLoad method.
override func viewDidLoad() { super.viewDidLoad() let refreshControl = UIRefreshControl() refreshControl.addTarget(self, action: #selector(sortArray), for: .valueChanged) self.refreshControl = refreshControl }
Every time the refresh control is pulled the UIControlEvent.ValueChanged event is triggered, which will call the sortArray method. Let's implement this method.
@objc func sortArray() { let sortedAlphabet = alphabet.reversed() for (index, element) in sortedAlphabet.enumerated() { alphabet[index] = element } tableView.reloadData() refreshControl?.endRefreshing() }
First the array is reversed with the reverse function. Then enumerate through the reversed array will be enumerated, where the reversed array will be loaded into the original alphabet array. The Table View is reloaded to update the contents and the RefreshControl animation is ended.
Build and Run, Pull-refresh a few times and the sorting order of the rows in the table view will be reversed.

You can download the source code of the IOSPullToRefreshTutorial at the ioscreator repository on Github
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK