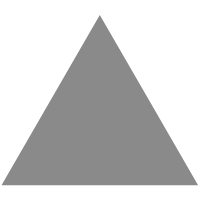
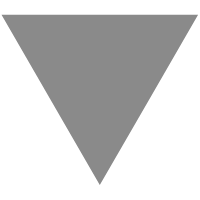
JavaFX TableView
source link: https://www.tuicool.com/articles/hit/77nuIba
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
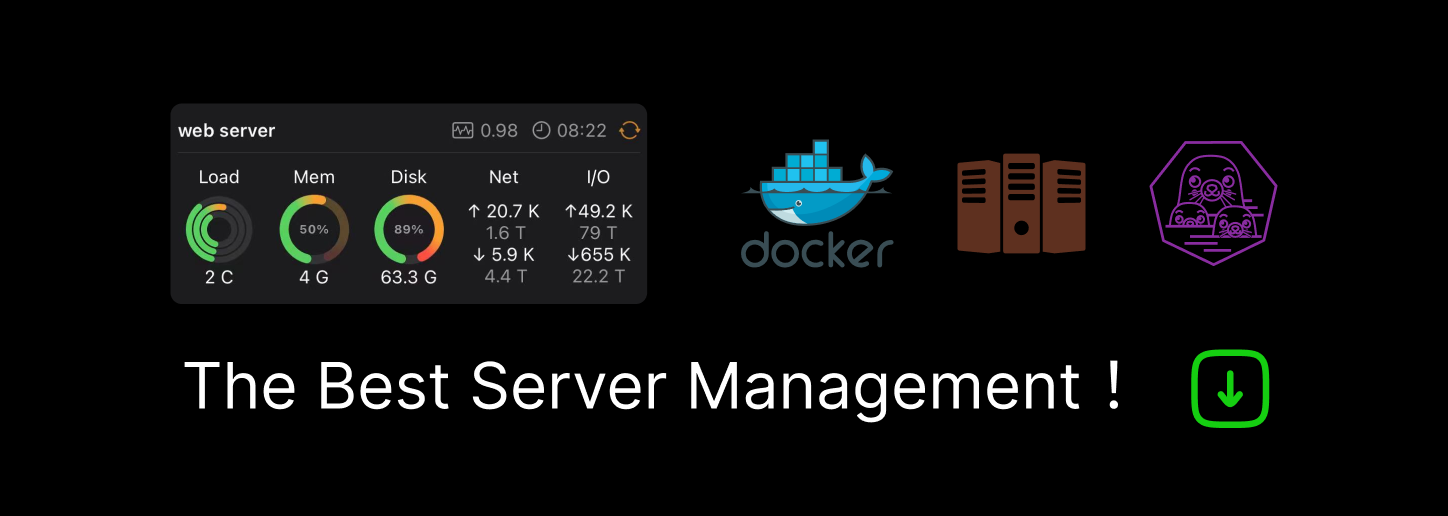
The JavaFX TableView enables you to display table views inside your JavaFX applications. The JavaFX TableView is represented by the class javafx.scene.control.TableView
. Here is a screenshot of a JavaFX TableView
:

JavaFX TableView Example
Here is a full, but simple JavaFX TableView
code example:
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.TableColumn; import javafx.scene.control.TableView; import javafx.scene.control.cell.PropertyValueFactory; import javafx.scene.layout.VBox; import javafx.stage.Stage; public class TableViewExample extends Application { public static void main(String[] args) { launch(args); } @Override public void start(Stage primaryStage) { TableView tableView = new TableView(); TableColumn<String, Person> column1 = new TableColumn<>("First Name"); column1.setCellValueFactory(new PropertyValueFactory<>("firstName")); TableColumn<String, Person> column2 = new TableColumn<>("Last Name"); column2.setCellValueFactory(new PropertyValueFactory<>("lastName")); tableView.getColumns().add(column1); tableView.getColumns().add(column2); tableView.getItems().add(new Person("John", "Doe")); tableView.getItems().add(new Person("Jane", "Deer")); VBox vbox = new VBox(tableView); Scene scene = new Scene(vbox); primaryStage.setScene(scene); primaryStage.show(); } }
Here is the Person
class used in this example:
public class Person { private String firstName = null; private String lastName = null; public Person() { } public Person(String firstName, String lastName) { this.firstName = firstName; this.lastName = lastName; } public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } }
Create a TableView
In order to use a JavaFX TableView
component you must first create a TableView
instance. Here is an example of creating a JavaFX TableView
instance:
TableView tableView = new TableView();
Add TableColumn to the TableView
Having created a TableView
you need to add one or more TableColumn
instances to the TableView
instance. A TableColumn
represents a vertical column of values. Each value is displayed on its own row, and is typically extracted from the list of objects being displayed in the TableView
. Here is an example of adding two TableColumn
instances to a JavaFX TableView
instance:
TableView tableView = new TableView(); TableColumn<String, Person> firstNameColumn = new TableColumn<>("First Name"); firstNameColumn.setCellValueFactory(new PropertyValueFactory<>("firstName")); TableColumn<String, Person> lastNameColumn = new TableColumn<>("Last Name"); lastNameColumn.setCellValueFactory(new PropertyValueFactory<>("lastName"));
TableColumn Cell Value Factory
A TableColumn
must have a cell value factory set on it. The cell value factory extracts the value to be displayed in each cell (on each row) in the column. In the example above a PropertyValueFactory
is used. The PropertyValueFactory
factory can extract a property value (field value) from a Java object. The name of the property is passed as a parameter to the PropertyValueFactory
constructor, like this:
PropertyValueFactory factory = new PropertyValueFactory<>("firstName");
The property name firstName
will match the getter getter method getFirstName()
of the Person
objects which contain the values are displayed on each row.
In the example shown earlier, a second PropertyValueFactory
is set on the second TableColumn
instance. The property name passed to the second PropertyValueFactory
is lastName
, which will match the getter method getLastName()
of the Person
class.
Add Data to TableView
Once you have added TableColumn
instances to the JavaFX TableView
, you can add the data to be displayed to the TableView
. The data is typically contained in a list of regular Java objects (POJOs). Here is an example of adding two Person
objects (class shown earlier in this JavaFX TableView tutorial) to a TableView
:
tableView.getItems().add(new Person("John", "Doe")); tableView.getItems().add(new Person("Jane", "Deer"));
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK