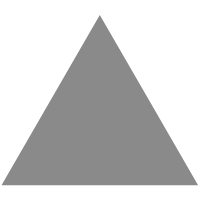
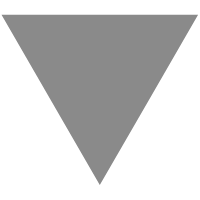
GitHub - AlanCheen/Flap: Flap is a library that makes RecyclerView.Adapter much...
source link: https://github.com/AlanCheen/Flap
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
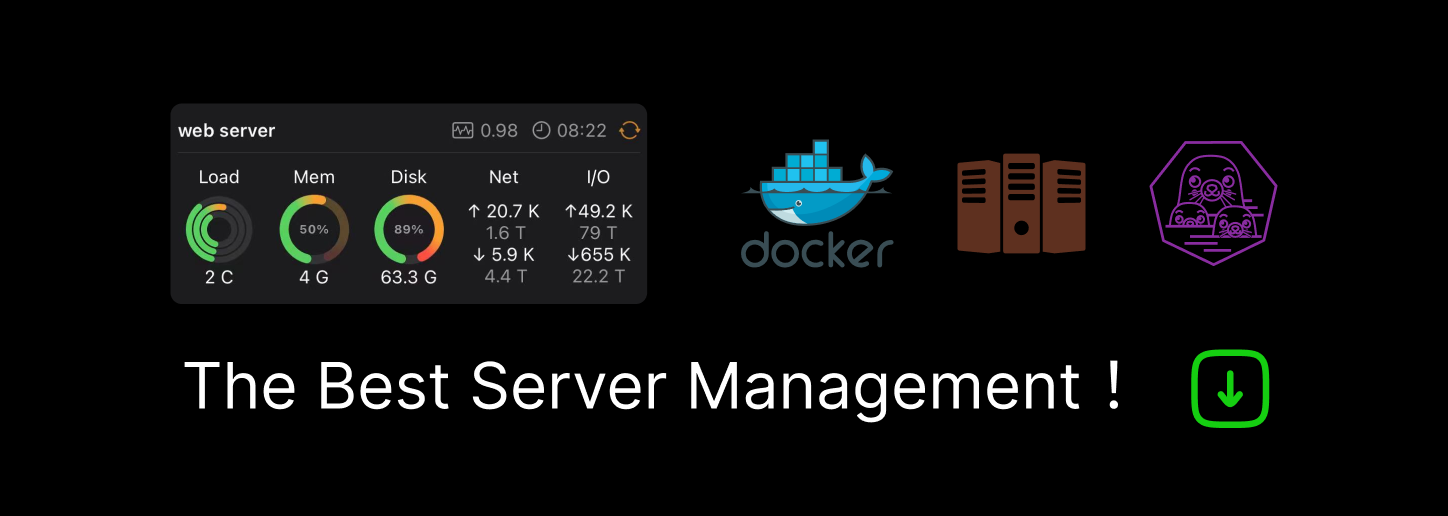
README.md
Flap
What is Flap
Flap
is a library that makes RecyclerView.Adapter
much more easier to use , by keeping you from writing boilerplate codes and providing lots advance features , especially when you have to support lots of different type items.
Getting Started
Integrate Flap
Add the latest Flap
to your dependencies:
dependencies {
implementation 'me.yifeiyuan.flap:flap:$lastest_version'
}
Usage
Step 1 : Create a model class :
public class SimpleTextModel { @NonNull public String content; public SimpleTextModel(@NonNull final String content) { this.content = content; } }
Step 2 : Create a FlapItem
and a LayoutItemFactory
:
FlapItem
is the base ViewHolder
that Flap
is using internally.
Here is a sample :
public class SimpleTextItem extends FlapItem<SimpleTextModel> { private static final String TAG = "SimpleTextItem"; private TextView tvContent; public SimpleTextItem(final View itemView) { super(itemView); tvContent = findViewById(R.id.tv_content); } @Override protected void onBind(@NonNull final SimpleTextModel model, @NonNull final FlapAdapter adapter, @NonNull final List<Object> payloads) { tvContent.setText(model.content); } public static class Factory extends LayoutItemFactory<SimpleTextModel, SimpleTextItem> { @Override protected int getLayoutResId(final SimpleTextModel model) { return R.layout.flap_item_simple_text; } } }
Step 3 : Register LayoutItemFactory
and create your FlapAdapter
Create your FlapAdapter
and register the Factory
, setup the data by the way :
//register your ItemFactory to Flap Flap.getDefault().register(new SimpleTextItem.Factory()); FlapAdapter adapter = new FlapAdapter(); List<Object> models = new ArrayList<>(); models.add(new SimpleTextModel("Android")); models.add(new SimpleTextModel("Java")); models.add(new SimpleTextModel("Kotlin")); //set your models to FlapAdapter adapter.setData(models); recyclerView.setAdapter(adapter);
NOTE: Just register Factory one time is enough , so you can register your Factories in your custom Application.
Yeah , you are good to go!
More Advanced Features
Flap
adds some features for FlapItem
:
- Access a context directly by field
context
. - Call
findViewById()
directlly instead ofitemView.findViewById
when you want to find a view. - Override
onViewAttachedToWindow
&onViewDetachedFromWindow
so that you can do something like pausing or resuming a video.
Enable Lifecycle
By extending LifecycleItem
, a lifecycle-aware ViewHolder
, you can get the lifecycle callbacks : onResume
、onPause
、onStop
、onDestroy
by default , when you care about the lifecycle , FlapAdapter
binds the LifecycleOwner
automatically.
Releated methods :
-
FlapAdapter.setLifecycleEnable(boolean lifecycleEnable)
enabled by default -
FlapAdapter.setLifecycleOwner(@NonNull final LifecycleOwner lifecycleOwner)
AsyncListDiffer supported
Flap
provides a build-in adapter DifferFlapAdapter
that supports AsyncListDiffer
feature.
Change Log
Check Releases for details.
Feature List
- Support AsyncListDiffer feature;
- Support setup global RecycledViewPool;
- Support Lifecycle for FlapItem;
- Decouple RecyclerView.Adapter and ViewHolder's creating and binding logic.
Contribution
Any feedback would be helpful , thanks!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK