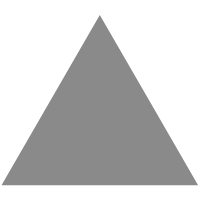
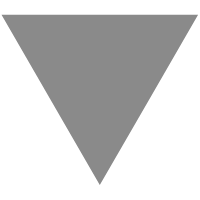
Beware of the findOne function
source link: https://www.tuicool.com/articles/hit/yq6vQnq
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
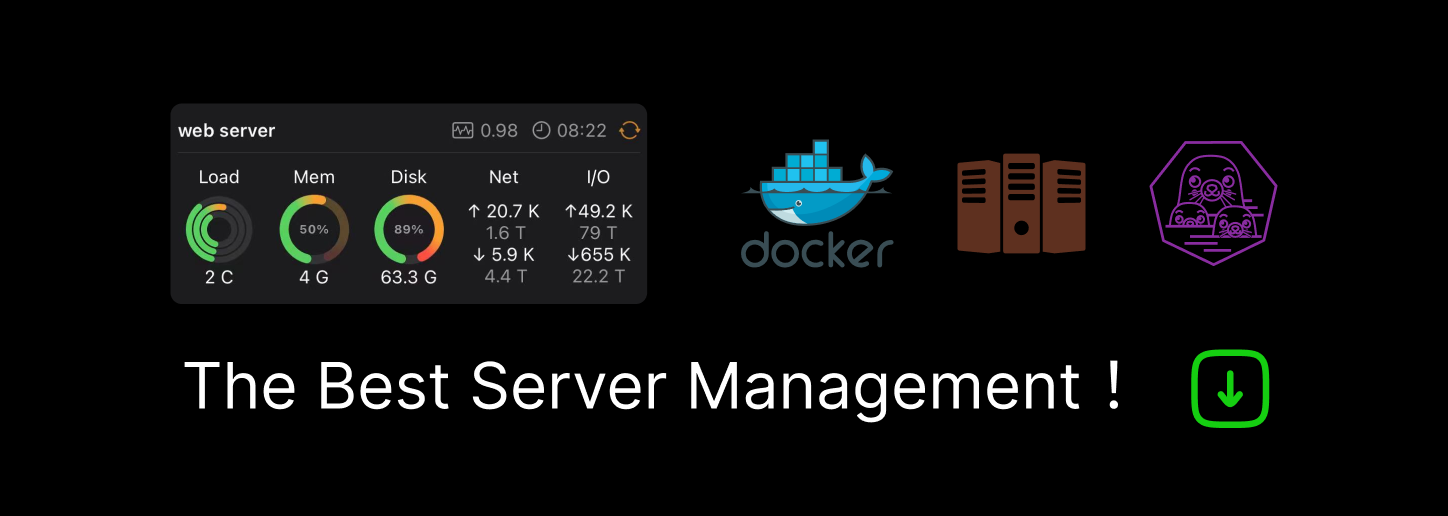
Hello, since my last post Easy Requests in NodeJS , I moved to the information security industry and started to study / investigate a lot about vulnerabilities in modern applications.
In this post, we will find how protect our web applications against NoSQL Injection
According with OWASP Top 10 - 2017 , the most frequent vulnerability in the last year was the A1:2017-Injection , which refers to the injection of a malicious code at a certain point in a vulnerable system, the most known injection is SQL Injection, through URLs, forms we can send malicious queries to the victim's database.
Nowadays, it is common to find systems that have an API to authenticate users and that use a non-relational database to store this information, a much used bank is Mongo .
In the example below, we used the NeDB bank which has a syntax very similar of Mongo.
controller
exports.login = async (req, reply) => { try { let { user, pass } = req.body let result = await findOne({user, pass}) return reply.code(200).send(result) } catch (e) { return reply.code(500).send({ success: false, result: 'user/pass not found' }) } }
db.findOne
async function findOne(query) { return new Promise((resolve, reject) => { db.findOne(query, (err, result) => { if (err) return reject(err) resolve({ success: true, result }) }) }) }
The login was made because the object we passed to findOne was a valid object, that is, both user and pass have values that actually exist in the database.
At the beginning of this post I commented on SQL Injection, but have you heard of NoSQL Injection? Not? Okay, you'll understand what this is, see the next function:
db.findOne(query, (err, result) => { if (err) return reject(err) resolve({ success: true, result }) })
Basically what this function does is a check in the database to know if there is any record with the values we passed to user && pass , note that I used the logical operator && (and ).
This does not make you think that if we pass at least the valid user and instead of the pass inform another validation that returns TRUE , will the function work?
Both Mongo and NeDB have filters that can be used in the queries in the database, for example the $gt , it is the equivalent of the relational operator " > ". Let's do a query using this filter instead of the password.
That is, we made a query in the database asking if it has a record with user "wubba" and that the value of pass is greater than "nothing", if there is a user with that name, of course the password will be greater than "nothing".
If we pass the same object {"$ gt": ""}
no user and pass , the bank will return the first record it has!
This shows us that the findOne function is dangerous if we do not create treatments for the values we pass to it, in this case we can validate if the information being informed is not an object.
To fix it we can use the following function
controller
exports.loginProtected = async (req, reply) => { try { let { user, pass } = req.body await isObject({ user, pass }) let result = await findOne({user, pass}) return reply.code(200).send(result) } catch (e) { return reply.code(500).send({ success: false, result: 'user/pass not found' }) } }
isObject
async function isObject(params) { return new Promise((resolve, reject) => { Object.keys(params).forEach((v, i) => { if (typeof params[v] === 'object') return reject(false) }) resolve(true) }) }
This case was reproduced using NeDB bank, but was also simulated using Mongo and Sails/Waterline , if you find in another bank, comment here to help someone else :wink:
Github Project https://github.com/nulldreams/nosql-pentest
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK