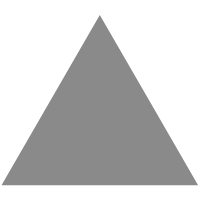
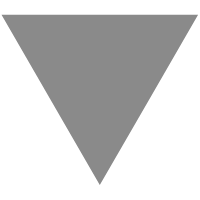
FormBuilder in Angular 6
source link: https://www.tuicool.com/articles/hit/rymMjuZ
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
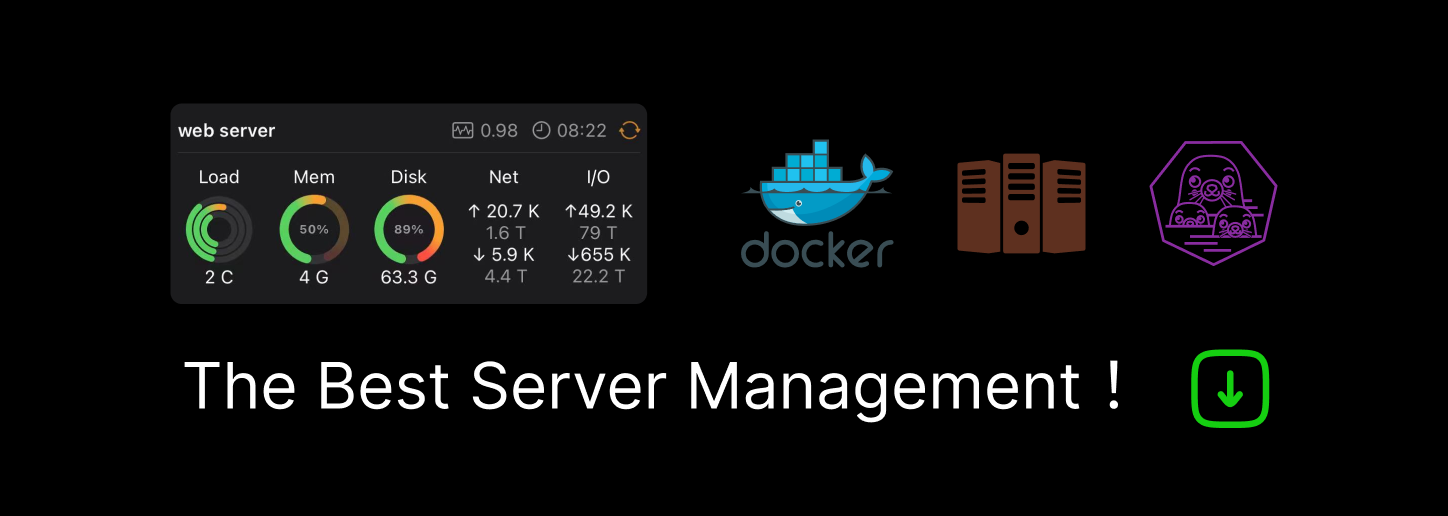
As a follow-up to my " New Application Journey " article, I wanted to focus on how FormBuilder in Angular improves the process of form-based aspects of your application, while introducing some reactive programming concepts along the way.
Recap
As a TL;DR
(too long, didn't read) to the original article, I wasn't happy with the application my mother-in-law was using for her very small business in the southeast section of the United States. So, I used her business needs to create a new application from scratch using Angular, MySQL, and the AWS environment. Since the application had a few forms to introduce, I was dreading the dated process I have been using — going all the way back to my introduction to AngularJS back in 2013.
Then, I noticed there was a FormBuilder in Angular, which used reactive programming concepts. This added a new layer of excitement for my new application journey.
What Is Reactive Programming?
According to Wikipedia, reactive programming is a declarative programming paradigm concerned with data streams and the propagation of change.
The Wikipedia page provides an example that I always recall when explaining reactive programming to new members of a current project. Typically, when using imperative programming the following line of code might be executed in a method or function:
a = b + c
Prior to reactive programming, the variable a
would be set to the sum of the current values of b
and c
at the time the line of code was executed. If later in the method, b
or c
changed, the value of a
would remain the same.
Reactive programming would consider each variable as a stream, with the concept that a will always equal the sum of b
and c
. Thus, if b
or c
changed, the value of a
would automatically change.
Angular FormBuilder
When building forms in the current version of Angular there are two options. The first is the typical template-based approach which relies on less explicit, unstructured data model, directives, and asynchronous predictability. The other is a reactive approach which relies on a more explicit, structured data model, functions, and synchronous predictability.
Since I had used the template approach for quite some time, I was excited to try the FormBuilder in a reactive mode.
A Simple Example
The following screenshot was shared in my original " New Application Journey " article:
This is a simple form, which has required fields for Property Name, Sales Price, and Commission amount. Since this screen shot was created, we removed the titleDate
and only now have a closingDate
. The closingDate
field is also required, but have a default value that can only be changed and not removed.
I needed to setup the FormBuilder object in Angular.
The constructor of my class included an inject of the FormBuilder
class, as shown below:
constructor(private formBuilder: FormBuilder, ...) { this.createForm(); }
When the constructor fires, the createForm()
method is called, which establishes the propertyForm
object:
private createForm(): void { this.propertyForm = this.formBuilder.group({ property: this.formBuilder.group({ propertyName: ['', Validators.required], closingDate: '', agentSales: '', lastName: '', agentInitials: '', salesPrice: ['', Validators.required], county: '', exemptAmount: '', baseTax: '', surtax: '', totalSalesTax: '', commission: ['', Validators.required], profit: '', totalAgentAmount: '', referralName: '', referralAmount: '' }), closingDate: ['', Validators.required], }); }
My propertyForm
includes a property object, which matches my DTO and a closingDate
object — which is used for the NgbDateStruct
implementation for the Closing Date field.
To initialize a new property on the form, I called a private method to establish the default values:
private createNewProperty(): void { const today = new Date(); this.property = new Property(); this.property.propertyName = null; this.property.closingDate = today.getTime(); this.property.salesPrice = null; this.property.commission = null; this.closingDate = { year: today.getFullYear(), month: today.getMonth() + 1, day: today.getDate() }; this.propertyForm.setValue({property: this.property, closingDate: this.closingDate});
When I need to push values into the FormBuilder
object, I use the setValue()
or patchValue()
methods. When I want to retrieve values from the FormBuilder
object, I use the value attributes on the this.propertyForm
object.
The biggest challenge I ran into with this form was getting the this.closingDate
values into the this.property.closingDate
attribute. The former is a JSON object with attributes for year, month, and day, while the latter is a Date()
object.
Looking Ahead
This article is a continuation of a multi-part series that I am putting together regarding my new application journey to providing a better application experience for my mother-in-law. Below, is a list of the current and planned articles, if you are interested in reading more:
-
FormBuilder in Angular 6 (this article)
-
The Challenge of the Commission Report (coming soon)
-
Getting CI/CD in place (coming soon)
-
What I Learned After Initial Deployment (coming soon)
Have a really great day!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK