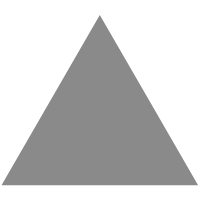
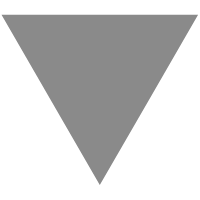
JavaScript Classes
source link: https://www.tuicool.com/articles/hit/ERziqmm
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
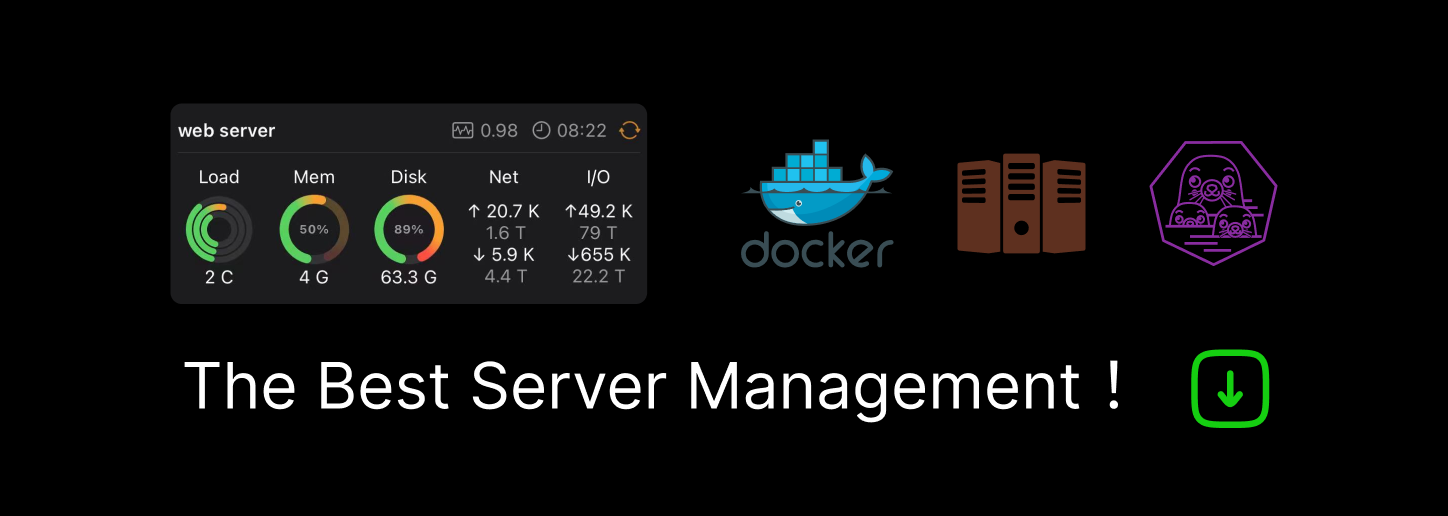
What is a JavaScript class?
A JavaScript class
is a function, which can be defined just as you would a function expression or function declaration. The class
syntax has two components:
-
class declaration
class Car {}
-
class expression unnamed
let Car = class {}
-
class expression named
let Car = class Toyota {}
class names should start with a capital letter
To declare a class, you use the class
keyword with the name of the class ("Car"). Class expressions can be named ("Toyota") or unnamed("Car"). The name given to a class expression is local to the class's body.
The class
function uses the constructor
and new
keywords to create templates for objects to be created. Using the class
function has benefits. Write less code, avoid typos, better readability, avoid repeating yourself(DRY).
Constructor
The constructor method, which resides inside a class is used to create and initialize an object created with a class
. You can only have one constructor method per class
. The constructor builds your object based on predefined criteria you set. See the below example,
class Car{ constructor(make, model, color) { this.make = make; this.model = model; this.color = color; } }
When I call this class
later the arguments I pass to it will be the make, model, and color for my Car object. The object that will be created from this will look like the example below,
const camry = new Car('Toyota', 'Camry', 'Blue'); console.log(camry); //Car {make:"Toyota", model: "Camry", color: "Blue"}
New
The new
operator creates an instance of a user-defined object type or of one of the built-in object types that has a constructor function. The new
operator essentially says hey create a new instance of this class
which we built above. The constructor
tells the new
operator, to use the arguments that were pass in to build the object.
conclusion
Using the class
function helps to create objects. What if we had to make 20 different cars that all had different colors and years! Not only would it take a while to create, we would have sooooo many lines of code to look through if we wanted to updated one.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK