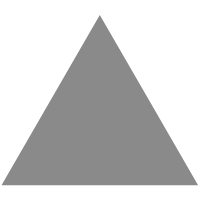
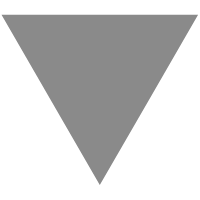
Learning Kotlin: Destructuring
source link: https://www.tuicool.com/articles/hit/6z6Zb2Q
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
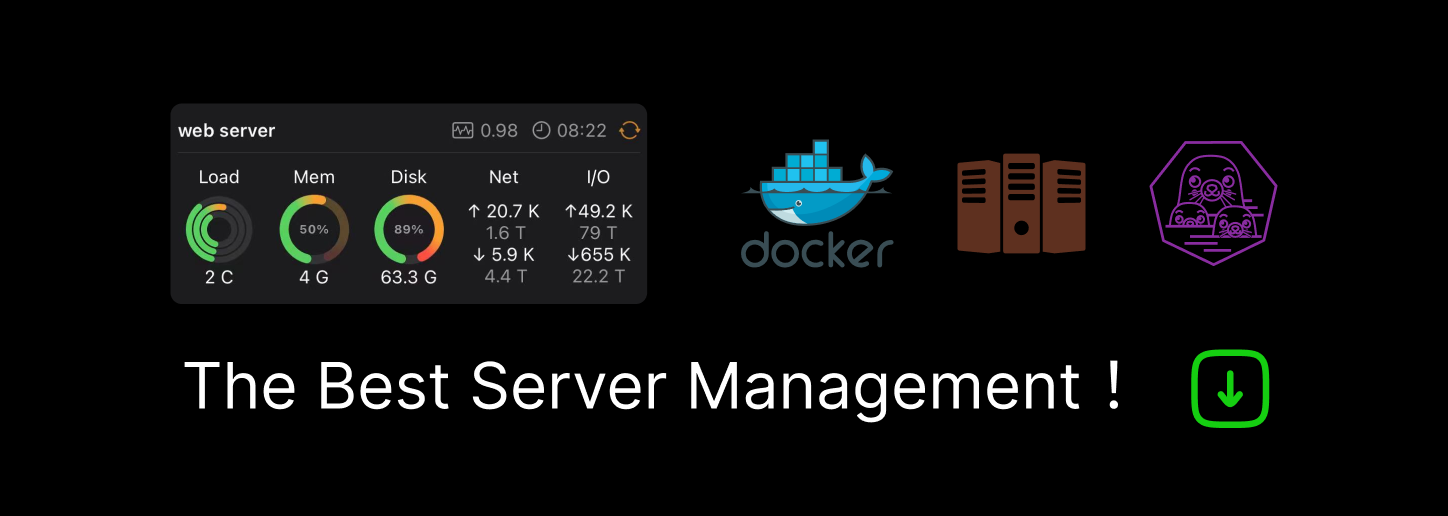
Learning a new language seems to be an experience that you undertake to:
- Change jobs
- Because your boss made you do it
- Because you are a "nerd"
The thing I forget each time I learn a new language is that the act of learning a new language helps me become a better software developer in my own primary language (the secret fourth option). Going through Kotlin has been a similar experience, and nothing jumped out more than object destructuring.
The simple use for object destructuring is to be able to do so in a single line and assign multiple variables from an object. Let's look at this example:
data class Person(val firstName: String, val surname: String, val age: Int) fun name(person: Person) { println("Hi ${person.firstName}") } fun name2(person: Person) { println("Hi ${person.firstName} ${person.surname}") } fun main(args:Array<String>) { val frank = Person("Frank", "Miller", 61) val alan = Person("Alan", "Moore", 64) name(frank) name2(frank) name(alan) name2(alan) }
In each of the name
and name2
, I am working with the Person
object, but all I want are the names. I never care about the age of the people.
We could add a function now, which pulls out just the strings we want and modify everything else to work with JUST the data it needs:
data class Person(val firstName: String, val surname: String, val age: Int) fun name(firstName: String) { println("Hi $firstName") } fun name2(firstName: String, surname: String) { println("Hi $firstName $surname") } fun print(person: Person) { val (firstName, surname) = person name(firstName) name2(firstName, surname) } fun main(args:Array<String>) { val frank = Person("Frank", "Miller", 61) val alan = Person("Alan", "Moore", 64) print(frank) print(alan) }
Line 12 is where the magic happens — that is the Object Destructuring
. Rather than having two lines where we assign a variable to firstName
and surname
, we can assign them both in one line so long as they are wrapped in parenthesis and match the names of the properties of the object.
So, why is this useful for other languages? This is because, in JavaScript, you have the same thing! The only difference is {
and }
rather than parenthesis, and since learning it in Kotlin, I've found that I use it in my main more, too.
Happy learning!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK